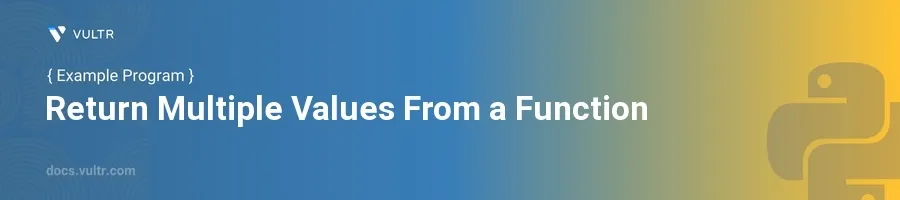
Introduction
Functions in Python are capable of returning multiple values, a feature that can significantly simplify and enhance the functionality of your programs. Utilizing this ability allows for cleaner code and the straightforward handling of multiple outputs from a single function call.
In this article, you will learn how to effectively harness this powerful feature in Python through practical examples. Discover how to return multiple values from a function and how to use these values in your code.
Returning Multiple Values From a Function
Using Tuples to Return Multiple Values
Define a function that performs multiple computations and returns the results as a tuple.
Call the function and directly unpack the values into multiple variables.
pythondef get_stats(numbers): min_val = min(numbers) max_val = max(numbers) average_val = sum(numbers) / len(numbers) return min_val, max_val, average_val # Example usage stats = get_stats([10, 20, 30, 40, 50]) min_number, max_number, average_number = get_stats([10, 20, 30, 40, 50]) print(f"Min: {min_number}, Max: {max_number}, Avg: {average_number}")
This function
get_stats
calculates the minimum, maximum, and average of a list of numbers. It returns these three values enclosed in a tuple. When called, the results can be immediately unpacked into three variables which can then be used independently.
Using Dictionaries to Return Named Values
Create a function that computes various statistics and returns them as a dictionary where each result has a named key.
Retrieve and use the individual results using their keys.
pythondef compute_metrics(data): length = len(data) total = sum(data) average = total / length return {'length': length, 'total': total, 'average': average} # Example usage metrics = compute_metrics([10, 20, 30, 40, 50]) print(f"Data Length: {metrics['length']}, Total Sum: {metrics['total']}, Average: {metrics['average']}")
In this example, the function
compute_metrics
performs computations to determine the length, total, and average of a dataset. The results are returned as a dictionary with appropriately named keys. This approach makes it very clear what each returned value represents, improving code readability.
Using Multiple Return Statements for Conditional Outputs
Define a function that evaluates conditions and returns different sets of values depending on the outcome.
Use the returned multiple values based on the condition.
pythondef process_data(value): if value > 50: return value, "High" else: return value, "Low" # Example usage number, category = process_data(75) print(f"Number: {number}, Category: {category}")
Here, the
process_data
function checks if the passed value is greater than 50, and returns the value along with a category string either "High" or "Low". This example shows how multiple return values can be conditionally managed within a function.
Conclusion
Returning multiple values from a function in Python can make your functions more versatile and your code more expressive. Whether you use tuples, dictionaries, or conditional multiple return statements, you can handle multiple outputs effectively. Explore these techniques in your projects to simplify the handling of various outputs from your functions, ensuring your code base remains clean and efficient.
No comments yet.