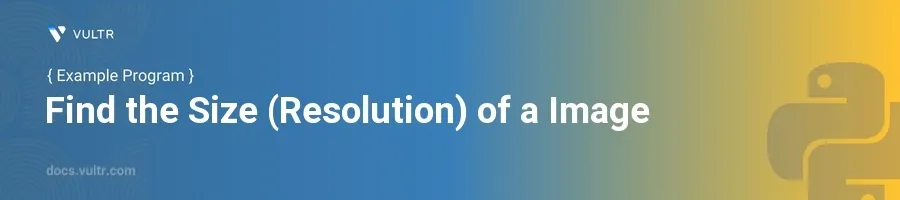
Introduction
Determining the size or resolution of an image is a fundamental task in many image processing applications. Being able to extract these attributes means you can dynamically modify, display, or analyze image data based on its dimensions. Python, equipped with powerful libraries like PIL (Pillow), makes this task straightforward.
In this article, you will learn how to get the image size in Python. Explore how to use the Pillow library to accomplish this with clarity and ease. Whether you need to get the image dimensions or check the resolution of an image in Python, practical examples that demonstrate reading and manipulating image properties below will help you understand the concept easily.
Finding Image Resolution with Pillow in Python
Pillow is the friendly PIL fork and an efficient library for opening, manipulating, and saving many different image file formats in Python. Using Pillow, you can get the image dimensions and extract the width and height of images easily. Here, focus on obtaining the resolution of images in various formats.
Install Pillow Library
Before diving into the code, ensure that the Pillow library is installed on your system. Here's how to install it using pip:
pip install Pillow
Basic Example to Get Image Size in Python
Import the
Image
module from thePIL
library.Open an image using
Image.open()
.Retrieve image size using the
size
attribute.pythonfrom PIL import Image # Load an image image = Image.open('path/to/your/image.jpg') # Get the size of the image width, height = image.size print(f"The image resolution is: {width}x{height}")
This code snippet opens an image and uses the
size
attribute, which returns a tuple containing the width and height of the image. This tuple is then unpacked intowidth
andheight
, which are printed in the console. Theimage.size
attribute is the most suitable to get the image dimensions in Python.
Get Size of Multiple Images in Python
To handle multiple images, iteratively process each image inside a directory and retrieve their sizes.
Import necessary modules.
Define a directory containing images.
Loop through each file, load the image, and print its size.
pythonimport os from PIL import Image # Directory containing images dir_path = 'path/to/your/directory' # List all files in the directory for file_name in os.listdir(dir_path): # Construct full file path file_path = os.path.join(dir_path, file_name) # Open and find the image size with Image.open(file_path) as img: print(f"{file_name}: {img.size}")
This code lists all files in a directory and processes each one through the
Image.open()
method. It retrieves the size of each image and prints the filename alongside its resolution. This approach is ideal when you want to check the image size in Python across multiple files.
Get Image Size from URLs in Python
In some cases, you might want to retrieve the size of an image hosted online without downloading it entirely.
Import necessary modules, including
requests
.Use
requests.get()
to stream the image.Open the image directly from bytes.
pythonimport requests from PIL import Image from io import BytesIO # URL of the image url = 'https://example.com/path/to/image.jpg' # Fetch the image response = requests.get(url, stream=True) response.raw.decode_content = True # Open the image with Image.open(response.raw) as img: print(f"Online image size: {img.size}")
This snippet fetches an image from a URL using
requests
with streaming enabled to avoid downloading the entire image. The image is then opened directly from the raw data stream usingImage.open()
. This method is useful when you want to get the image size from a URL without saving the file locally.
Conclusion
Extracting the size or resolution of images using Python and Pillow is both efficient and easy to encapsulate within functional parts of programs. Whether dealing with local files, multiple images, or online sources, Pillow provides a consistent and straightforward interface for image manipulation. Apply the concepts learned here to enhance applications that rely on dynamic image resizing, checks, or processing. By mastering these techniques, you can check image sizes in Python efficiently and maintain clean, readable, and effective code for managing image data.
No comments yet.