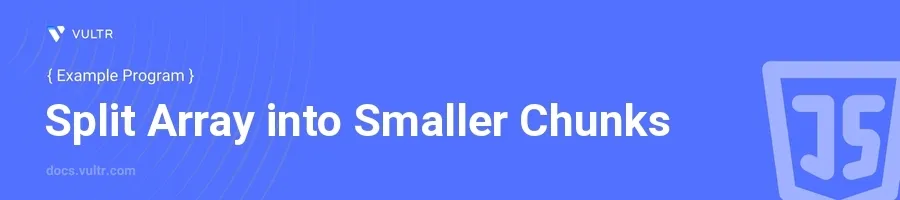
Introduction
Splitting an array into smaller chunks is a common task in software development, often required when processing large datasets in manageable segments or when optimizing performance for data handling tasks. This functionality can be particularly useful in web development, where managing data efficiently can impact performance and user experience significantly.
In this article, you will learn how to effectively split an array into smaller chunks using JavaScript. Various methods will be explored, ranging from traditional loops to more modern approaches using ES6 features like Array.from()
and slice()
. By the end of this guide, you will have a robust toolkit of techniques for handling arrays in your JavaScript projects.
Traditional Loop-based Approach
Split Arrays with a For Loop
Initiate an empty array to hold the chunks.
Use a for loop to iterate over the array and slice it into the desired chunk size.
javascriptfunction splitArrayTraditional(arr, chunkSize) { let result = []; for (let i = 0; i < arr.length; i += chunkSize) { let chunk = arr.slice(i, i + chunkSize); result.push(chunk); } return result; }
This function takes two parameters:
arr
, the original array, andchunkSize
, the size of each chunk. The for loop iterates over the array, slicing it from the current indexi
toi + chunkSize
, and then pushes this chunk into the result array. This method continues until the entire array is processed.
Example Usage
Create an array of numbers.
Call the
splitArrayTraditional
function.javascriptlet numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; let chunks = splitArrayTraditional(numbers, 4); console.log(chunks);
This snippet splits the
numbers
array into chunks of 4 items each, resulting in the output:[[1, 2, 3, 4], [5, 6, 7, 8], [9, 10]]
.
Modern ES6 Approach
Using Array.from() to Create Chunks
Utilize
Array.from()
to generate a new array based on the number of chunks.Inside
Array.from()
, use theslice()
method to create each chunk based on the current index multiplied by the chunk size.javascriptfunction splitArrayES6(arr, chunkSize) { return Array.from({ length: Math.ceil(arr.length / chunkSize) }, (_, i) => arr.slice(i * chunkSize, i * chunkSize + chunkSize) ); }
Array.from()
creates a new array from an array-like or iterable object. Here, it's used to create the correct number of chunks by determining the array length divided by the chunk size, rounded up usingMath.ceil()
. The second argument is a mapping function that takes each chunk out of the original array.
Example Usage
Define an array of elements.
Invoke
splitArrayES6
and examine the output.javascriptlet items = ["apple", "banana", "cherry", "date", "fig", "grape"]; let itemChunks = splitArrayES6(items, 2); console.log(itemChunks);
In this example, the
items
array is divided into chunks of 2, producing[['apple', 'banana'], ['cherry', 'date'], ['fig', 'grape']]
.
Conclusion
Mastering the technique of splitting arrays into smaller chunks in JavaScript is essential for handling large datasets and improving data processing tasks effectively. Whether you choose the traditional looping technique or prefer the concise ES6 methods, both approaches provide robust solutions for segmenting arrays. Adapt these methodologies to fit the specific needs of your projects, ensuring efficient data management and optimal performance in your web applications. These skills not only enhance your coding toolkit but also open up possibilities for more complex and responsive web development solutions.
No comments yet.