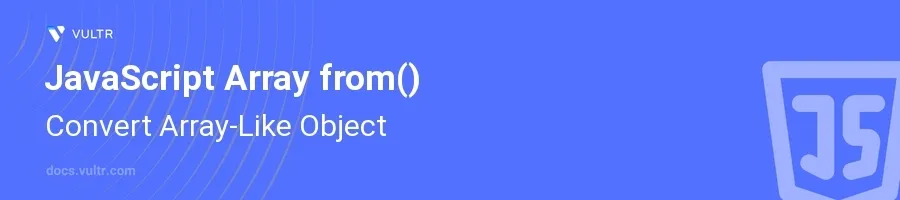
Introduction
The Array.from()
method in JavaScript allows converting array-like or iterable objects into a new array instance. This functionality bridges the gaps between array-like structures (such as DOM NodeList objects) and true JavaScript arrays, enabling the full application of JavaScript's array methods.
In this article, you will learn how to effectively use the Array.from()
method to transform array-like objects into true arrays. Explore various practical scenarios, including converting a string to an array, managing NodeList objects from the DOM, and utilizing iterables or mapping functions.
Basic Usage of JavaScript Array.from() Method
Converting a String to an Array
Define a string you wish to convert.
Use
Array.from()
to convert the string into an array.javascriptconst str = "Hello"; const strArray = Array.from(str); console.log(strArray);
This code converts the string
"Hello"
into an array with each character as a separate element. The output would be['H', 'e', 'l', 'l', 'o']
.
Converting JavaScript NodeList to Array using Array.from()
Consider a NodeList obtained by using a method like
document.querySelectorAll()
.Convert this NodeList into an array using
Array.from()
.javascriptconst nodes = document.querySelectorAll('div'); const nodesArray = Array.from(nodes); console.log(nodesArray);
The snippet above converts a NodeList of
div
elements into an array, allowing you to use array methods likemap()
,filter()
orreduce()
on it.
Advanced Uses of JavaScript Array.from()
Working with Iterables
Define an iterable object, such as a Set.
Convert the iterable into an array using
Array.from()
.javascriptconst mySet = new Set([1, 2, 3]); const myArray = Array.from(mySet); console.log(myArray);
This converts a
Set
object into an array. The resulting array will be[1, 2, 3]
.
Using a Map Function
Utilize
Array.from()
to not only convert but also transform elements in one go.javascriptconst baseNumbers = [1, 2, 3, 4]; const squares = Array.from(baseNumbers, x => x * x); console.log(squares);
The above example demonstrates converting an array of numbers into their squares using a map function.
Array.from()
here acts on an array-like input and maps each elementx
tox * x
, resulting in the array[1, 4, 9, 16]
.
Conclusion
The Array.from()
function in JavaScript is a highly versatile tool for converting array-like and iterable objects into true arrays. This method simplifies the processing of non-array collections by providing a seamless way to transform and manipulate these structures with standard array operations. Whether dealing with strings, NodeList objects, or other iterable collections, Array.from()
efficiently standardizes them into arrays, enhancing code manageability and functionality. Through the examples and techniques discussed, grasp the utility of this method to streamline your JavaScript development tasks.
No comments yet.