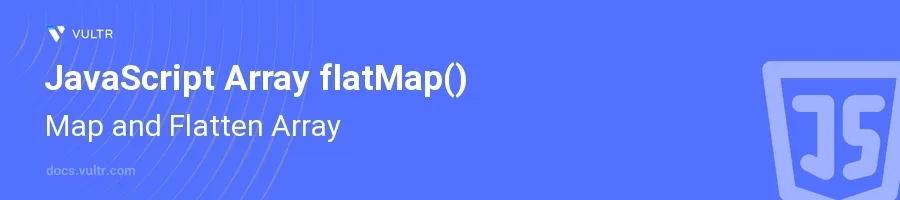
Introduction
The flatMap()
method in JavaScript is a powerful array function that combines the capabilities of map()
and flat()
into a single operation. This method first maps each element using a mapping function, then flattens the result into a new array. It's particularly useful for dealing with nested arrays or when you need to perform a transformation and flattening step in one go.
In this article, you will learn how to effectively utilize the flatMap()
method in JavaScript. Explore various examples that demonstrate how to transform and flatten arrays simultaneously, simplifying the processing of array data significantly.
Understanding flatMap()
Basic Usage of flatMap()
Start with a simple array that includes nested arrays.
Use the
flatMap()
method to both map and flatten the array in a single step.javascriptlet arr = [1, 2, [3, 4]]; let flatMappedArr = arr.flatMap(x => [x * 2]); console.log(flatMappedArr);
This example demonstrates basic usage where
flatMap()
helps to double the values and flattens the array. Note that it only flattens up to one level deep.
Handling Nested Arrays
Create a more complex array structure that includes multiple levels of nesting.
Map each element to modify it, then flatten the result with
flatMap()
.javascriptlet nestedArr = [1, [2, [3, 4]]]; let result = nestedArr.flatMap(x => Array.isArray(x) ? x : [x]); console.log(result);
Here,
flatMap()
checks if an element is an array and, if not, wraps it in an array before flattening. This effectively flattens the array one level deeper than a simple map and flat would, but it does not handle deeper nesting automatically.
Combining with Other Array Methods
Understand how
flatMap()
can be integrated with other array methods such asfilter()
.Chain
flatMap()
withfilter()
to achieve more complex transformations.javascriptlet students = [ {name: 'Alice', scores: [60, 70, 80]}, {name: 'Bob', scores: [80, 85, 90]} ]; let scores = students.flatMap(student => student.scores.filter(score => score >= 80)); console.log(scores);
In this context, each student's scores are filtered to include only those 80 or above, then
flatMap()
flattens the arrays of scores into a single array.
Common Uses of flatMap()
Data Transformation
Apply
flatMap()
in scenarios requiring transformation and flattening in data processing tasks.Consider an example handling data transformation:
javascriptlet books = [ {title: "JavaScript", authors: ["Author A", "Author B"]}, {title: "CSS", authors: ["Author C"]} ]; let authorsList = books.flatMap(book => book.authors.map(author => author.toUpperCase())); console.log(authorsList);
This snippet takes an array of books, each with a list of authors, maps over each author to convert their name to uppercase and flattens the result into a single list of authors.
Streamlining Code
- Use
flatMap()
to simplify and streamline code that deals with arrays within arrays. - Recognize the reduction in code complexity and enhancement in readability when using
flatMap()
over separatemap()
andflat()
operations.
Conclusion
The flatMap()
function in JavaScript is an essential tool for working with nested arrays, particularly when both mapping and flattening are required. By combining these two operations into a single method, it simplifies the code and improves performance in specific scenarios. Utilize this function whenever you're faced with nested arrays and need a concise way to transform and flatten the data. With the strategies outlined in this article, harness the power of flatMap()
to write cleaner, more efficient JavaScript code.
No comments yet.