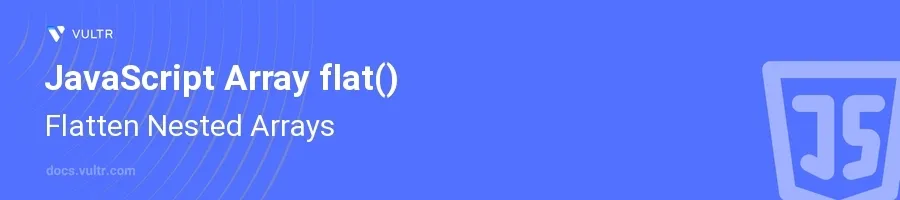
Introduction
The flat()
method in JavaScript provides a straightforward approach for flattening nested arrays, transforming them into a single-dimensional array. This is particularly useful when dealing with data structures where handling multiple levels of nested arrays can become cumbersome and complex in terms of access and manipulation.
In this article, you will learn how to proficiently use the flat()
method across various scenarios. Explore how this method can simplify interactions with multi-dimensional arrays by understanding its basic usage and adapting it for deeper nested arrays.
Understanding Flat()
The flat()
method in JavaScript is called on an array and returns a new array where all sub-array elements concatenated into it recursively up to the specified depth.
Basic Usage of flat()
Define an array that contains nested arrays.
Use the
flat()
method without specifying a depth to flatten the array one level deep.javascriptconst nestedArray = [1, [2, [3, 4]]]; const flatArray = nestedArray.flat(); console.log(flatArray);
This code takes a nested array and flattens it one level. The output will be
[1, 2, [3, 4]]
.
Specify the Depth of Flattening
Determine the depth of nested arrays you want to flatten.
Use the
flat()
method and pass the depth as a parameter to flatten arrays to the desired level.javascriptconst deepNestedArray = [1, [2, [3, [4, 5]]]]; const flatDeepArray = deepNestedArray.flat(2); console.log(flatDeepArray);
In the example above, two levels of nested arrays are flattened. The resulting array will be
[1, 2, 3, [4, 5]]
.
Handling Empty Arrays
Recognize that empty arrays within nested structures are removed when flattened.
Observe how the
flat()
method impacts arrays with empty sub-arrays.javascriptconst arrayWithEmpty = [1, [], [2, [3]]]; const result = arrayWithEmpty.flat(); console.log(result);
This will produce
[1, 2, [3]]
as empty arrays are removed in the process.
Flattening Completely
Use the
Infinity
parameter withflat()
to flatten arrays regardless of depth.Apply this method to a highly nested array structure.
javascriptconst veryDeepArray = [1, [2, [3, [4, [5]]]]]; const fullyFlatArray = veryDeepArray.flat(Infinity); console.log(fullyFlatArray);
This flattens the array across all levels, resulting in
[1, 2, 3, 4, 5]
.
Conclusion
The flat()
method in JavaScript is a versatile tool that significantly eases the handling of nested arrays. By allowing you to specify the depth of flattening, it provides flexibility in how deep you want to go in the array structure. This functionality is essential for data manipulation where the depth of nested data varies. Employ the techniques discussed to enhance how you manage and interact with multi-dimensional arrays in your projects, ensuring that your code is cleaner and more maintainable.
No comments yet.