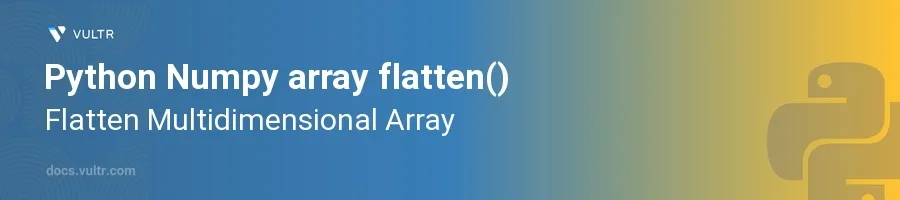
Introduction
The flatten()
method in Numpy is an invaluable tool when dealing with multidimensional arrays in Python, especially when you need to convert these arrays into a one-dimensional format. This transformation is fundamental in various scientific computing tasks, ranging from data preprocessing in machine learning to simplifying matrix operations.
In this article, you will learn how to efficiently utilize the flatten()
method in Python to manage and manipulate multidimensional arrays. Explore practical examples of transforming complex arrays into simpler, single-dimensional arrays and understand the options available within the flatten()
function to customize the flattening process.
Whether you need to flatten a 2D array in Python, flatten an array of arrays, or convert a NumPy array to a list, this guide covers all essential techniques.
##Understanding the flatten() Function in Python
Basic Usage of flatten()
Import the Numpy library.
Create a multidimensional array.
Apply the
flatten()
method to flatten the 2D array in Python.pythonimport numpy as np multi_array = np.array([[1, 2], [3, 4], [5, 6]]) flat_array = multi_array.flatten() print(flat_array)
This code creates a simple 2D array and uses
flatten()
to transform it into a 1D array, producing the output[1 2 3 4 5 6]
. Theflatten()
method on a NumPy array is the easiest approach to flatten 2D arrays in Python.
Flatten with Different Orders
Recognize that
flatten()
can alter the way the array is read during the flattening process using theorder
parameter.Experiment with both 'C' (row-major) and 'F' (column-major) orders to flatten a matrix in Python.
pythonC_flat_array = multi_array.flatten(order='C') F_flat_array = multi_array.flatten(order='F') print("Row-major flattening:", C_flat_array) print("Column-major flattening:", F_flat_array)
With 'C' order, the array is flattened row-wise, and with 'F' order, it's column-wise. The output will display the difference:
[1 2 3 4 5 6]
for 'C' and[1 3 5 2 4 6]
for 'F'. When working with a NumPy 3D array, you can use the same method to convert it into a 1D array. However, if you need to flatten a 3D array into a 2D array, consider usingreshape()
instead.
Practical Applications of flatten()
Preparing Data for Machine Learning
Understand the importance of data reshaping in machine learning data preprocessing.
Flatten a more complex multidimensional array to create feature vectors using
numpy.flatten()
.pythondata_matrix = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]]) feature_vector = data_matrix.flatten() print("Feature vector:", feature_vector)
The
flatten()
method converts the 3D array into a 1D feature vector, crucial for training models in machine learning frameworks. Flattening images in Python is another common application for this method. This method efficiently handles the transformation if you need to flatten a structured NumPy array.
Simplifying Operations in Scientific Computing
Realize that flattening can simplify operations such as applying functions uniformly across data.
Use
flatten()
in a scenario where uniform function application is necessary, such as flattening an inhomogeneous array in NumPy.pythontemperatures = np.array([[30, 35], [20, 25], [40, 45]]) normalized_temps = np.log(temperatures.flatten()) print("Log of temperatures:", normalized_temps)
Here, applying the logarithm function becomes straightforward after flattening the temperature matrix. This is a great example of flattening a NumPy array for mathematical operations. Additionally, when working with a NumPy vectorized 2D array,
flatten()
ensures all elements are processed efficiently.
Conclusion
The flatten()
function in Python's Numpy library is a critical tool for data processing, enabling the easy transformation of multidimensional arrays into single-dimensional arrays. It supports various configurations to suit particular requirements and aids in data preparation for machine learning, simplifying matrix operations, and more. By mastering its various applications and settings, like row-major or column-major order, you can transform complex datasets into simplistic and manageable forms and enhance both the scalability and flexibility of your computational tasks. Whether flattening a NumPy array of arrays, converting a NumPy array to a list in Python, or flattening an n-dimensional array into a 1D array, the flatten()
function remains an indispensable utility for data manipulation.
No comments yet.