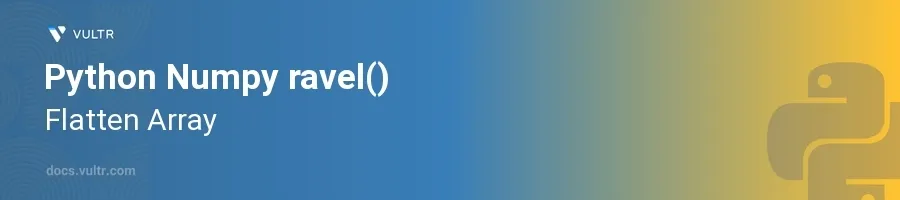
Introduction
The ravel()
function from the NumPy library in Python is essential for transforming multi-dimensional arrays into a contiguous flattened one-dimensional array. This capability is particularly handy in data processing and manipulation tasks where such transformation is required for algorithm compatibility or data restructuring purposes.
In this article, you will learn how to use the ravel()
function to flatten arrays effectively. Explore different scenarios where this function can be applied to manage data efficiently, illustrating its behavior with real-world examples.
Using ravel() to Flatten Arrays
Flatten a Two-Dimensional Array
Create a two-dimensional array.
Apply the
ravel()
function.pythonimport numpy as np two_d_array = np.array([[1, 2, 3], [4, 5, 6]]) flattened_array = np.ravel(two_d_array) print(flattened_array)
This code converts
two_d_array
, a 2x3 matrix, into a flattened one-dimensional array. Theravel()
function processes the elements in row-major (C-style) order by default, yielding the output[1 2 3 4 5 6]
.
Preserve Array Order with 'F'-Order
Understand that the ordering of elements can be controlled using the
order
parameter.Create an array and specify the
order
parameter as 'F' (column-major order).Use the
ravel()
function to flatten the array.pythonmulti_d_array = np.array([[1, 2, 3], [4, 5, 6]]) flattened_F_order = np.ravel(multi_d_array, order='F') print(flattened_F_order)
With the 'F' order parameter in
ravel()
, the function processes the array's elements in column-major order. For our array, this results in[1 4 2 5 3 6]
as opposed to the default row-major order.
Handling Higher-Dimensional Arrays
Prepare to work with arrays that have more than two dimensions.
Apply
ravel()
to convert it into a one-dimensional array.pythonthree_d_array = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]]) flat_three_d = np.ravel(three_d_array) print(flat_three_d)
In this example, a three-dimensional array is successfully transformed into a single flattened array
[1 2 3 4 5 6 7 8]
, demonstrating howravel()
efficiently handles more complex structures.
Conclusion
The ravel()
function is a versatile tool in NumPy for flattening arrays into a one-dimensional structure. Whether dealing with simple two-dimensional arrays or more complex ones, utilizing this function ensures that data manipulation becomes more straightforward. By mastering ravel()
and understanding its parameters like order
, you enhance data preprocessing tasks and promote clearer, more concise data handling code. Use these examples as a foundation to integrate ravel()
into various data processing workflows efficiently.
No comments yet.