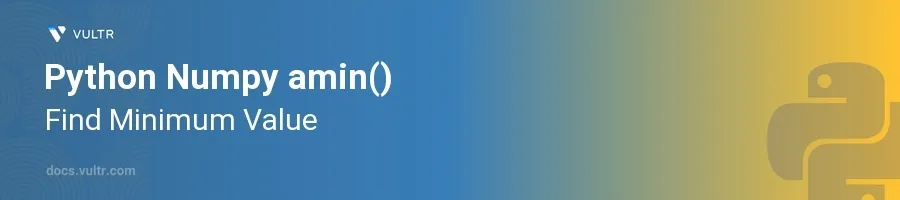
Introduction
The amin()
function in Python's NumPy library is a handy tool for finding the minimum value of an array or along a specific axis of the array. This is particularly useful in data analysis, statistics, and machine learning, where you may need to determine the smallest element quickly and efficiently from a large dataset or a multidimensional array.
In this article, you will learn how to effectively use the amin()
function to find minimum values across various scenarios and data structures. You will explore different examples that demonstrate the power and versatility of this function with both single-dimensional and multi-dimensional arrays.
Understanding amin() in Single-Dimensional Arrays
Finding Minimum Value in an Array
Import the NumPy library.
Create a single-dimensional array.
Apply the
amin()
function to find the smallest element.pythonimport numpy as np array = np.array([23, 42, 7, 15, 39]) min_value = np.amin(array) print(min_value)
This code snippet finds the minimum value
7
in the array. The functionnp.amin()
processes each element to determine the smallest one.
Working with Multi-Dimensional Arrays
Minimum Value Across a Whole Array
Define a two-dimensional array.
Use
amin()
to determine the smallest value across all elements.pythonimport numpy as np matrix = np.array([[5, 12, 1], [7, 9, 11], [18, 21, 20]]) overall_min = np.amin(matrix) print(overall_min)
The above code checks across all nested arrays and correctly identifies
1
as the smallest number in the entire matrix.
Minimum Value Along an Axis
Specify the axis along which you wish to find the minimum value.
Apply
amin()
with theaxis
parameter.pythonaxis0_min = np.amin(matrix, axis=0) axis1_min = np.amin(matrix, axis=1) print("Minimum per column:", axis0_min) print("Minimum per row:", axis1_min)
In this example:
axis=0
finds the minimum values among all the columns ([5, 9, 1]
).axis=1
finds the minimum values among all the rows ([1, 7, 18]
).
Conclusion
The amin()
function in NumPy is a powerful tool for extracting the minimum value from an array or along a specific array axis. It caters perfectly to the needs in fields like data analysis where quick and efficient computation of the smallest values in datasets is crucial. Understanding how to utilize this function with different configurations and dimensions extends your data manipulation capabilities and makes your analyses more robust and efficient. By integrating these techniques into your workflow, you facilitate better decision-making and data interpretation processes.
No comments yet.