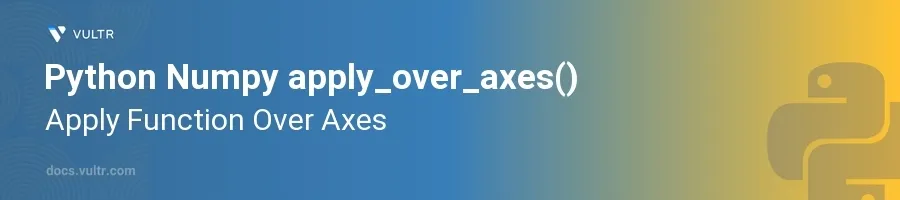
Introduction
The apply_over_axes()
function in the Numpy library is a potent tool that helps apply a function iteratively over given axes of an array. This approach is particularly beneficial for tasks involving data manipulation and transformation across multiple dimensions in an array. It streamlines processes that would otherwise require explicit loops, thus enhancing code efficiency and clarity.
In this article, you will learn how to utilize the apply_over_axes()
function in different scenarios. Delve into the mechanics of assigning functions to specific axes of multidimensional arrays and learn the essential tweaks needed to get the desired outcomes.
Basics of apply_over_axes()
Understanding the Function Signature
- Recognize that the syntax for
apply_over_axes()
isnumpy.apply_over_axes(func, a, axes)
. - Identify that
func
is any function that you want to apply,a
is the ndarray, andaxes
is the tuple of axes over which to applyfunc
.
Simple Application on a 2D Array
Start with a basic function, such as the sum, to apply over rows and columns.
Define a 2D array and apply
apply_over_axes()
to sum across a specific axis.pythonimport numpy as np def sum_func(arr, axis=0): return np.sum(arr, axis=axis) array_2d = np.array([[1, 2], [4, 5]]) result = np.apply_over_axes(sum_func, array_2d, axes=(1,)) print(result)
This code sums up the elements across axis 1 (columns for a 2D array). The function
sum_func
performs this sum, andapply_over_axes()
applies it across 2D array defined.
Advanced Usage with Multi-dimensional Arrays
Work with a 3D Array
Consider more complex data, like a three-dimensional array.
Apply a function over multiple axes and observe different results based on axis selection.
pythonarray_3d = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]]) # Example function to calculate the maximum def max_func(arr, axis=0): return np.max(arr, axis=axis) result = np.apply_over_axes(max_func, array_3d, axes=(1,2)) print(result)
The function
max_func
is designed to find the maximum element, applied here over axes 1 and 2 simultaneously. This yields the maximum per each selection along those two axes in thearray_3d
.
Custom Function Application
Design a custom function to apply specific operations not available by default in Numpy.
Use
apply_over_axes()
to apply this custom function on complex array structures.pythondef custom_operation(arr, axis=0): return np.mean(arr, axis=axis) * 0.5 custom_result = np.apply_over_axes(custom_operation, array_3d, axes=(2,)) print(custom_result)
This example applies a custom operation (mean followed by a multiplication) over axis 2. It demonstrates the flexibility of
apply_over_axes()
when used with user-defined functions.
Conclusion
The apply_over_axes()
function in Numpy is vital for efficient, readable data manipulation across multiple dimensions of an array. Its ability to accept any function and apply it over specified axes can drastically reduce the complexity of operations performed on large datasets. Whether using built-in Numpy functions or tailored user-defined scenarios, apply_over_axes()
optimizes and simplifies multidimensional array manipulations, promoting cleaner and more efficient code workflows. Utilize this function in diverse data analytical applications to streamline your data processing tasks.
No comments yet.