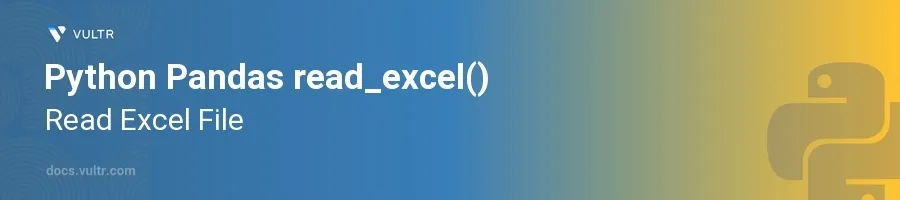
Introduction
The read_excel()
function from the Pandas library is a convenient and powerful tool for importing Excel files into a DataFrame, enabling data manipulation and analysis in Python. This function supports reading from both .xls
and .xlsx
formats and offers various parameters to customize the import process according to specific requirements.
In this article, you will learn how to effectively harness the read_excel()
function to load Excel data into Python. Explore how to handle different data types, skip rows, use columns, and manipulate sheets within a workbook, enhancing your data analysis capabilities.
Basics of read_excel()
Reading a Basic Excel File
Import the Pandas library and use the
read_excel()
function.Specify the path to the Excel file.
pythonimport pandas as pd df = pd.read_excel('path/to/your/file.xlsx') print(df.head())
This code loads an Excel file into a DataFrame
df
and prints the first few rows using thehead()
method. Ensure the file path is correct to avoid any file-not-found errors.
Specifying Sheet Name
By default,
read_excel()
loads the first sheet of the Excel workbook.Specify a particular sheet by name or index.
pythondf = pd.read_excel('file.xlsx', sheet_name='Sheet2') print(df.head())
Here,
sheet_name='Sheet2'
instructs Pandas to load a sheet named 'Sheet2' from the Excel file. You can also use the sheet index, wheresheet_name=0
would be the first sheet.
Advanced Options in read_excel()
Selecting Specific Columns
Use the
usecols
parameter to specify the columns to load.pythondf = pd.read_excel('file.xlsx', usecols=['A', 'C', 'E']) print(df.head())
The
usecols
parameter filters the input so only the named columns—'A', 'C', and 'E'—are read from the Excel file.
Skipping Rows
Skip rows at the beginning of the file using the
skiprows
parameter.This is useful for ignoring header information that you do not need.
pythondf = pd.read_excel('file.xlsx', skiprows=4) print(df.head())
Setting
skiprows=4
skips the first four rows of the Excel file. Adjust the number based on the number of header rows in your document.
Handling Data Types and Missing Values
Specifying Data Types
Use the
dtype
parameter to define the data types of columns explicitly.This control ensures data types are correctly interpreted.
pythondf = pd.read_excel('file.xlsx', dtype={'EmployeeID': int, 'Salary': float}) print(df.dtypes)
This snippet explicitly sets the 'EmployeeID' column as integers and the 'Salary' column as floats, which can be crucial for subsequent data handling and computations.
Filling Missing Values
Handle missing data by specifying a value to replace any blanks or missing entries.
Employ the
na_values
parameter to treat certain values as missing.pythondf = pd.read_excel('file.xlsx', na_values=['NA', '?', '']) print(df.isna().sum())
In the above code,
na_values
identifies 'NA', '?', and empty strings as NaN in the DataFrame, which can then be counted or replaced as required.
Conclusion
The read_excel()
function in Pandas is a versatile tool for importing Excel data into Python. It supports a variety of functionalities that accommodate diverse data structures and requirements. Mastering this function enables you to seamlessly transition from Excel spreadsheets to powerful Python data frames, unlocking extensive data analysis and manipulation features. Implement these techniques in your next data project to maintain robust and efficient data workflows.
No comments yet.