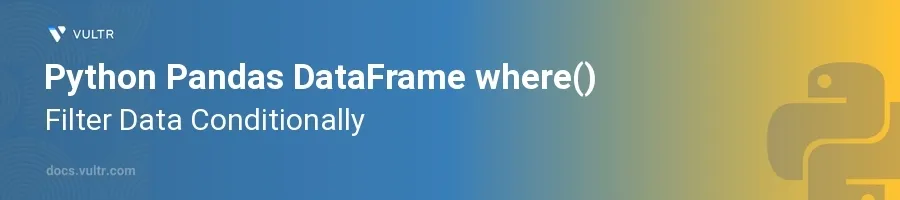
Introduction
The where()
function in Pandas is a versatile tool designed to filter data in a DataFrame based on a condition. This function is particularly useful in data analysis and preprocessing, where you need to selectively alter or extract data based on specific criteria without modifying the original DataFrame structure.
In this article, you will learn how to effectively utilize the where()
function in Python's Pandas library to filter and manipulate data conditionally. Discover various applications of the function, understand how it differs from similar methods, and explore how to apply it to both single and multiple conditions.
Understanding the where() Function
Basic Usage of where()
Import the Pandas library and create a DataFrame.
Apply the
where()
function to filter data based on a condition.pythonimport pandas as pd data = {'A': [10, 20, 30, 40], 'B': [5, 15, 25, 35]} df = pd.DataFrame(data) filtered_df = df.where(df['A'] > 20)
The DataFrame
filtered_df
will retain the rows where column 'A' has values greater than 20. Rows that do not meet the condition will have NaN (Not a Number) in all columns.
Maintaining Original Data Shape
Retain the shape of the original data by setting
other
to a default value.Apply the
where()
function with theother
parameter.pythonresult = df.where(df['A'] > 20, other=0)
In this case, rows where the condition is False will replace all values with 0 instead of NaN. This is useful when you need non-null values across the entire DataFrame for subsequent data processing steps.
Advanced Usage of where()
Combining Multiple Conditions
Define a DataFrame with more complex data.
Use multiple conditions in the
where()
method by combining them with logical operators.pythondf = pd.DataFrame({'A': range(1, 6), 'B': range(10, 0, -2)}) result = df.where((df['A'] > 1) & (df['B'] < 8))
This will filter the DataFrame under the combined conditions where 'A' is greater than 1 and 'B' is less than 8. The result will include NaN where conditions are not met.
Applying Column-Specific Conditions
Use the
where()
function to apply conditions specific to columns.Incorporate dictionary comprehension for dynamic column-specific criteria.
pythonconditions = {'A': 3, 'B': 5} result = df.where(df > pd.Series(conditions))
Each column checks against its corresponding value in the
conditions
dictionary. This method is scalable and flexible, especially when dealing with many columns having varied criteria.
where() vs. mask()
Understanding how where()
contrasts with another similar Pandas function, mask()
, is essential for precise data filtering:
where()
keeps original values where the condition is True; otherwise, it replaces with NaN or specified value.mask()
does the opposite: it keeps the values where the condition is False.
Example of using mask()
Apply
mask()
to the same DataFrame to see the inverse effect.pythonmasked_df = df.mask(df['A'] > 20, other=0)
This code places 0s where the condition is True, unlike
where()
which does so where the condition is False.
Conclusion
The where()
function in Pandas provides a powerful way to filter and manipulate data frames based on conditional logic, maintaining the integrity and structure of the original data. By mastering this function, along with understanding its relationship to similar methods like mask()
, you enhance your data manipulation capabilities in Python. Use this knowledge to perform nuanced data filtering and transformation tasks efficiently, empowering your data analysis projects with precision and ease.
No comments yet.