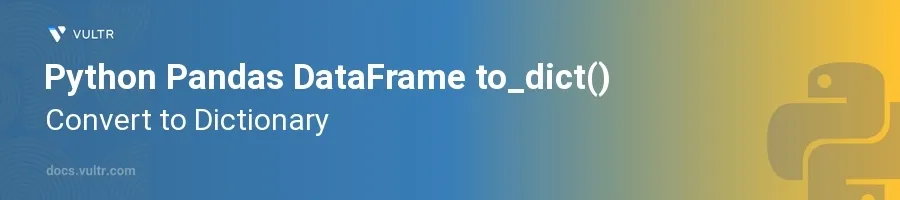
Introduction
Working with data in Python often involves manipulating and transforming data structures, and one of the most common transformations is converting a Pandas DataFrame into a dictionary. The Pandas library provides powerful tools for data manipulation, one of which is the to_dict()
method, which allows for easy conversion of DataFrame objects into dictionaries. This can be particularly useful when you need to interface with APIs or perform operations that are more naturally handled with dictionaries.
In this article, you will learn how to efficiently utilize the to_dict()
method to transform a DataFrame into different dictionary formats based on your requirements. You will explore various orientations for the conversion and understand how each format structures the data, providing flexibility depending on the use case.
Understanding DataFrame to Dictionary Conversion
Basic Conversion with Default Parameters
Begin by creating a pandas DataFrame.
Use the
to_dict()
method without any arguments to perform a basic conversion.pythonimport pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'City': ['New York', 'Los Angeles', 'Chicago']} df = pd.DataFrame(data) dict_result = df.to_dict() print(dict_result)
This code outputs a dictionary where the keys are the column names and the values are dictionaries of row indices with corresponding cell values.
Specifying the orient
Parameter
The orient
parameter of the to_dict()
method defines the structure of the resulting dictionary. Each option provides a different format, and understanding these can help tailor the output to specific needs.
Convert Using 'list' Orientation
Set the
orient
parameter to'list'
.Observe how the DataFrame columns convert into dictionary keys associated with lists of column values.
pythondict_list_oriented = df.to_dict(orient='list') print(dict_list_oriented)
Here, each key in the dictionary represents a DataFrame column, and the value is a list of the column's entries, preserving order.
Convert Using 'records' Orientation
Adjust the
orient
parameter to'records'
.Examine the output where each row in the DataFrame becomes a separate dictionary in a list.
pythondict_records_oriented = df.to_dict(orient='records') print(dict_records_oriented)
In this format, the dictionary consists of a list of dictionaries, with each dictionary representing a row from the DataFrame, making it ideal for JSON serialization.
Convert Using 'index' Orientation
Change the
orient
option to'index'
.Check how the conversion results in a dictionary keyed by the DataFrame index.
pythondict_index_oriented = df.to_dict(orient='index') print(dict_index_oriented)
This option organizes the dictionary with DataFrame indices as keys, each mapping to a dictionary of column:value pairs for that particular row.
Convert Using 'split' Orientation
Choose
'split'
for theorient
parameter.Notice that the dictionary contains three keys: 'index', 'columns', and 'data'.
pythondict_split_oriented = df.to_dict(orient='split') print(dict_split_oriented)
This structure separates the indices, columns, and data into distinct elements, useful for re-constructing the DataFrame or for custom processing.
Using 'dict' Orientation (Default)
Use the
to_dict()
function with the defaultorient
which is'dict'
.Realize the standard dictionary structure output, where columns become dictionary keys.
python# This repeats the basic conversion example adding clarity about default orientation. dict_default_oriented = df.to_dict() # Equivalent to df.to_dict(orient='dict') print(dict_default_oriented)
This output is identical to the basic conversion shown initially, emphasizing the default behavior of the method.
Conclusion
The versatility of the to_dict()
method in converting a Pandas DataFrame to a dictionary is a powerful feature for Python data manipulation and analysis. By strategically selecting the orientation parameter, you adapt the data structure to fit various application requirements, from data serialization to direct manipulation. Experiment with different orientations depending on your context. Whether you are preparing data for a web application, a data science model, or simply transforming data for easier access, mastering this conversion process enhances your data handling capabilities. Through the examples and explanations provided, you are well-equipped to implement this method effectively in your projects.
No comments yet.