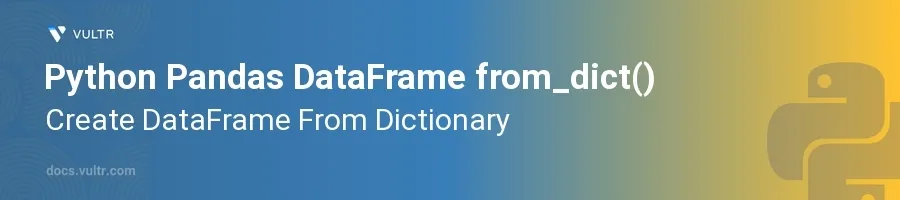
Introduction
The from_dict()
method in the Pandas library is an invaluable tool for transforming dictionary data into a structured DataFrame. This feature plays a crucial role in data manipulation and analysis, enabling users to easily convert collections of data organized as dictionaries into DataFrame objects that can be further manipulated and analyzed using Pandas' powerful data handling capabilities.
In this article, you will learn how to utilize the from_dict()
method effectively across various scenarios. Discover method's flexibility with different dictionary orientations and learn how to transform these varied dictionary formats into well-structured DataFrame objects.
Creating a Basic DataFrame from a Dictionary
Convert a Simple Dictionary Into DataFrame
Prepare a dictionary with coherent data.
Use
from_dict()
to convert the dictionary into a DataFrame.pythonimport pandas as pd data_dict = {'fruit': ['Apple', 'Banana', 'Cherry'], 'count': [12, 24, 30]} df = pd.DataFrame.from_dict(data_dict) print(df)
This code snippet takes the
data_dict
dictionary, where fruits and their respective counts are listed, and converts it into a DataFrame using Pandas'from_dict()
method. The resulting DataFrame will have fruits and counts as columns with corresponding values.
Handle a Dictionary with Nested Lists
Consider a scenario where each dictionary key has values in a nested list.
Convert this complex structure into a DataFrame using the same method.
pythondata_dict_complex = {'fruit': [['Apple', 'Red'], ['Banana', 'Yellow'], ['Cherry', 'Red']]} df_complex = pd.DataFrame.from_dict(data_dict_complex) print(df_complex)
Here, each fruit is associated with its color within a nested list. The
from_dict()
method still effectively creates a DataFrame, where each list becomes a separate row within the respective column.
Advanced DataFrame Construction
Specifying Columns While Creating DataFrame
Explicitly define which dictionary keys to use as DataFrame columns.
Create a DataFrame using only selected columns from the dictionary.
pythonselective_data_dict = {'fruit': ['Apple', 'Banana', 'Cherry'], 'count': [12, 24, 30], 'color': ['Red', 'Yellow', 'Red']} df_selective = pd.DataFrame.from_dict(selective_data_dict, columns=['fruit', 'color']) print(df_selective)
In this case, even though the dictionary contains three keys, the
columns
parameter in thefrom_dict()
method allows for the selection of specific columns. The resulting DataFrame contains only 'fruit' and 'color' columns.
Using Different Orientations
Understand that dictionaries can be oriented differently when using
from_dict()
.Utilize the
orient
parameter to specify how to interpret the dictionary structure.pythondict_orientation = {'row1': {'fruit': 'Apple', 'count': 12}, 'row2': {'fruit': 'Banana', 'count': 24}} df_oriented = pd.DataFrame.from_dict(dict_orientation, orient='index') print(df_oriented)
This snippet demonstrates how to use a dictionary that uses row labels as dictionary keys. By setting the
orient
parameter to'index'
, each key becomes a row in the DataFrame, with sub-dictionary keys as columns.
Conclusion
The from_dict()
method from the Pandas library provides a robust way to efficiently convert dictionaries into DataFrame objects, paving the way for more advanced data analysis and manipulation. Whether dealing with simple dictionary structures or more complex nested data, this method allows for flexible DataFrame creation that can be tailored to your specific data handling needs. Harness these techniques to enhance the structure and analysis capabilities of your Pandas workflows.
No comments yet.