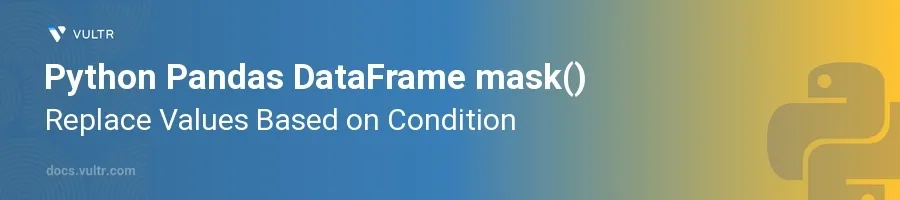
Introduction
The mask()
function in the powerful Pandas library offers a dynamic way to modify the contents of a DataFrame or Series by masking values based on a specified condition. This function selectively alters elements that satisfy a particular criterion, replacing them with another value or the result of a callable you specify. Ideal for data preprocessing and transformation, mask()
enhances data manipulation in Python.
In this article, you will learn how to efficiently use the mask()
function in various data manipulation scenarios. Discover how to apply conditions, integrate callable functions for dynamic replacements, and handle missing data within Pandas DataFrames.
Basic Usage of mask()
Applying a Simple Condition
Create a sample DataFrame.
Define a simple condition.
Use the
mask()
function to replace values meeting the condition.pythonimport pandas as pd df = pd.DataFrame({ 'A': range(1, 6), 'B': range(10, 0, -2) }) masked_df = df.mask(df > 3, 'Above 3') print(masked_df)
This code snippet masks entries greater than 3 in the DataFrame
df
by replacing them with the string 'Above 3'. It demonstrates the fundamental application ofmask()
—swapping out values that meet a certain criterion with a new value.
Masking with a Callable Function
Employ a function that depends on DataFrame values.
Utilize this function within
mask()
.pythondf = pd.DataFrame({ 'data': range(5) }) def calculate(value): return value * 2 if value > 2 else value masked_df = df['data'].mask(df['data'] > 2, calculate) print(masked_df)
Using a callable within
mask()
, this approach allows for dynamic calculation of the replacement values, where entries greater than 2 in the 'data' column are doubled.
Advanced Masking Techniques
Masking Based on External Criteria
Assume an external list to compare with DataFrame indices.
Apply
mask()
based on this external criterion.pythondf = pd.DataFrame({ 'A': range(5), 'B': list('abcde') }) external_criteria = [0, 2, 4] masked_df = df.mask(df.index.isin(external_criteria), 'Selected') print(masked_df)
Applying
mask()
with an index-wise condition can be particularly useful for cases where the inclusion criterion stems from external computations or datasets.
Combined Conditions
Combine multiple conditions using logical operators.
Replace values meeting these conditions.
pythondf = pd.DataFrame({ 'A': range(10), 'B': range(20, 30) }) masked_df = df.mask((df['A'] < 5) & (df['B'] > 25), 'Condition Met') print(masked_df)
This example combines two conditions before applying the mask, showcasing flexibility in handling more complex filtering requirements.
Using mask() with Missing Data
Handling NaN Values with Conditional Replacements
Work with a DataFrame containing NaNs.
Use
mask()
to replace NaN entries conditionally.pythondf = pd.DataFrame({ 'Values': [1, 2, None, 4], 'State': ['NY', 'NY', 'CA', 'CA'] }) masked_df = df['Values'].mask(df['Values'].isna(), df['Values'].fillna(0)) print(masked_df)
This scenario focuses on replacing NaN values based on the requirement to fill them with zeros only in certain conditions (demonstrated with simplicity here). The mask function is very versatile when handling missing data based on other columns or conditions in the DataFrame.
Conclusion
The mask()
function in Pandas is a robust tool for replacing DataFrame elements based on specific conditions. Whether you're dealing with basic replacements, dynamic function-based conditions, or complex masking logic, this function streamlines the process of data transformation in Python. By mastering the techniques discussed, you ensure that your data manipulation processes are both efficient and adaptable to varying analysis needs.
No comments yet.