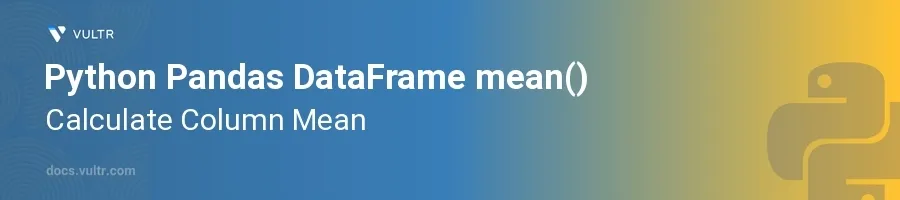
Introduction
The mean()
function in the Python Pandas library is designed to compute the mean, or average, of data within a DataFrame. As a fundamental statistical function, it is invaluable when analyzing large datasets to derive insights through average values, which can highlight trends and central tendencies in the data.
In this article, you will learn how to effectively utilize the mean()
function to calculate the mean of various columns in a DataFrame. You'll explore examples that demonstrate how to compute the average of numeric data and handle non-numeric or missing data to ensure accurate and meaningful outputs.
Calculating Mean of DataFrame Columns
Basic Usage of mean()
Start by importing the Pandas library and creating a DataFrame.
Apply the
mean()
function to calculate the mean of numeric columns.pythonimport pandas as pd data = { 'A': [1, 2, 3, 4, 5], 'B': [2, 3, 4, 5, 6], 'C': [3, 4, 5, 6, 7] } df = pd.DataFrame(data) column_mean = df.mean() print(column_mean)
The above snippet creates a DataFrame from a dictionary of lists. Applying
mean()
computes the average across each numeric column, resulting in a Series where each index corresponds to a column name from the DataFrame.
Computing Mean with Axis Option
Understand that the
mean()
function can compute along different axes.Use the
axis
parameter to direct the operation along rows instead of the default column calculation.pythonrow_mean = df.mean(axis=1) print(row_mean)
Setting
axis=1
changes the direction of mean calculation to operate across rows (horizontally) instead of the default column operation (vertically). Each row's mean is computed across all its numeric columns.
Handling Missing Data in Mean Calculation
Recognize that missing values can affect the mean calculation.
Use the
skipna
option to control how NaN values are treated.pythondata_with_nan = { 'A': [1, None, 3, 4, 5], 'B': [2, 3, 4, None, 6], 'C': [None, 4, 5, 6, 7] } df_with_nan = pd.DataFrame(data_with_nan) mean_without_nan = df_with_nan.mean(skipna=True) print(mean_without_nan)
By default,
skipna=True
ensures thatmean()
skips over any NaN values during computation. However, if you setskipna=False
, the function will return NaN for any column involving NaN values in its mean computation.
Specifying Columns for Mean Calculation
Determine if a mean calculation is required for specific columns rather than all columns.
Explicitly select the columns before applying the
mean()
function.pythonspecified_column_mean = df[['A', 'C']].mean() print(specified_column_mean)
Here, only the columns 'A' and 'C' are selected for mean calculation. This approach is useful when dealing with a DataFrame containing a mix of numeric and non-numeric types, or when you are only interested in a subset of all available data columns.
Conclusion
Mastering the mean()
function in Pandas empowers you to perform essential statistical analysis on your data. The ability to calculate average values efficiently can prove critical in data exploration and preprocessing stages of a data science project. By understanding how to harness different parameters such as axis
and skipna
, and by specifying the exact columns for mean computation, you ensure your analysis is both effective and precise. Make extensive use of these techniques in your numerical data analysis to maintain clarity and accuracy in your insights.
No comments yet.