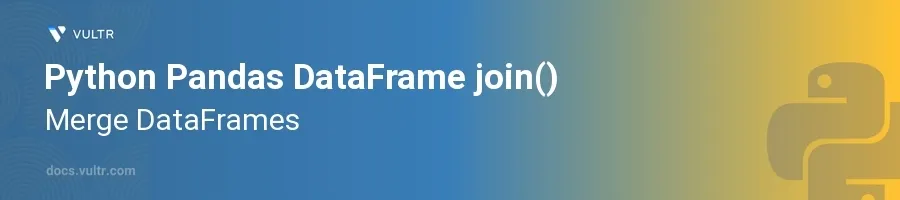
Introduction
Pandas joins, particularly through the join()
method, are essential in data wrangling and analytics, providing powerful ways to combine data from multiple DataFrame objects based on index or column alignment. Understanding how to effectively leverage this function can greatly enhance data manipulation and analysis capabilities in Python.
In this article, you will learn how to efficiently use the join() method in pandas to merge DataFrames. Gain insights into different types of joins, explore how to handle various merging scenarios, and see practical examples to solidify your understanding of these techniques.
Understanding DataFrame Join
Basics of join()
- Review that
join()
is a method for combining different DataFrame objects. - Note that by default,
join()
merges DataFrames on their indexes. - Recognize that
join()
allows joining on columns by setting theon
parameter.
Syntax and Parameters
Look at the basic syntax of the
join()
method:pythonDataFrame.join(other, on=None, how='left', lsuffix='', rsuffix='', sort=False)
This function includes several parameters:
- other: The DataFrame you want to join with.
- on: The column or index level names to join on in the calling DataFrame.
- how: Type of join to perform (left, right, inner, outer).
- lsuffix, rsuffix: Suffixes to use from left and right DataFrame columns when there is an overlap.
- sort: Sorts the resulting DataFrame by the join keys if
True
.
Join Types Explained
Default Left Join
Understand the default behavior is a left join.
Left joins include all rows from the left DataFrame and the matched rows from the right DataFrame.
Unmatched entries will have
NaN
in columns of the right DataFrame.pythonimport pandas as pd df1 = pd.DataFrame({'A': [1, 2, 3]}) df2 = pd.DataFrame({'B': [4, 5, 6]}, index=[1, 2, 3]) result = df1.join(df2) print(result)
Explanation:
df1
is joined withdf2
based on their indices.- Since the default join type is 'left', all indices of
df1
are included in the resulting DataFrame. Wherever the indices do not match,NaN
appears for the missing values.
Using Inner Join
Recall that an inner join returns only the common elements.
Useful in filtering out unmatched data points.
pythonresult_inner = df1.join(df2, how='inner') print(result_inner)
Explanation:
- Only indices present in both DataFrames are included in the result.
Exploring Outer and Right Joins
Outer join is used to get the union of keys from both frames.
Right join is similar to left but includes all entries from the right DataFrame.
pythonresult_outer = df1.join(df2, how='outer') print(result_outer) result_right = df1.join(df2, how='right') print(result_right)
Explanation:
- The outer join provides a complete set from both DataFrames.
- Right join prioritizes entries from the right DataFrame, filling with
NaN
for missing left side data.
Joining on Columns
Direct Column Join
Specify the
on
parameter to join on a DataFrame’s column.Ensure the column exists in the left DataFrame.
pythondf1 = pd.DataFrame({'Key': [1, 2, 3], 'Val': [7, 8, 9]}) df2 = pd.DataFrame({'Val': [10, 11, 12], 'Extra': [13, 14, 15]}) result = df1.join(df2.set_index('Val'), on='Val') print(result)
Explanation:
df2
is set to use 'Val' as its index temporarily for joining purposes.df1
uses its 'Val' column to align withdf2
.
Conclusion
The join()
function in pandas is a fundamental tool for merging DataFrames, crucial for effective data analysis and manipulation. By mastering various join types and understanding how to apply them in practical contexts, you streamline the process for merging data and extracting useful insights. Use these techniques to handle complex data alignment challenges efficiently and confidently in your data projects.
No comments yet.