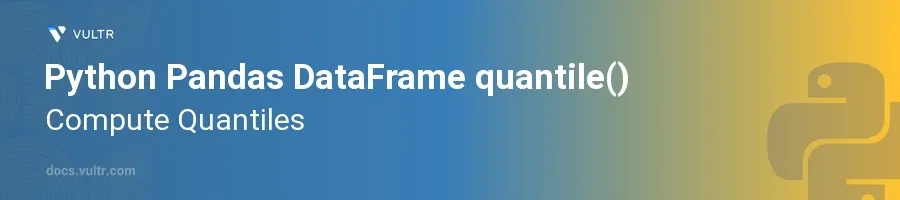
Introduction
Quantiles are vital statistics in data analysis, commonly used to understand the distribution and spread of data points. The quantile()
method in the Python Pandas library is a powerful tool for computing quantiles from data series or DataFrames, helping in effective data summarization and insight generation. Whether you're working in finance, science, or marketing, understanding how to leverage the quantile function is crucial for dealing with datasets.
In this article, you will learn how to harness the quantile()
method on Pandas DataFrames. Explore the flexibility of this function through various examples that reflect different scenarios such as handling missing data, applying it across different axes, and customizing quantile calculations.
Understanding the quantile() Method
Basic Quantile Calculation
Import the Pandas library and create a DataFrame.
Use the
quantile()
method to compute quantiles.pythonimport pandas as pd data = {'scores': [23, 45, 56, 78, 89, 100, 34, 55, 77, 88]} df = pd.DataFrame(data) quantile_50 = df['scores'].quantile(0.5) print(quantile_50)
This example calculates the 50th percentile (median) of the scores within the DataFrame. The
quantile()
method by default considers the numeric series and computes the median value, which is 66.5 in this scenario.
Computing Multiple Quantiles at Once
Understand how to request multiple quantiles in a single call.
Generate a list of desired quantiles and apply the
quantile()
method.pythonquantiles = df['scores'].quantile([0.25, 0.5, 0.75]) print(quantiles)
When passed a list of quantile values, the
quantile()
method returns a Series containing the results for each quantile. This results in finding the 1st quartile, median, and 3rd quartile (25th, 50th, and 75th percentiles).
Custom Quantiles and Non-Numeric Data Exclusion
Customize quantile computation beyond the common statistics.
Utilize the
quantile()
method on a DataFrame excluding non-numeric data.pythondata = {'scores': [56, 89], 'categories': ['large', 'small']} df = pd.DataFrame(data) custom_quantiles = df.select_dtypes(include=[float, int]).quantile([0.2, 0.4, 0.9]) print(custom_quantiles)
In this example, by using
select_dtypes()
, it's easy to exclude non-numeric columns before computing quantiles. Custom quantiles of 20%, 40%, and 90% are calculated from the numeric data available.
Advanced Usage of quantile()
Computing Quantiles Across Different Axes
Recognize the flexibility of the
quantile()
method in handling DataFrame dimensions.Compute quantiles across different axes of a DataFrame.
pythonmulti_data = { 'exam1': [56, 89, 78], 'exam2': [72, 91, 84], 'exam3': [88, 73, 77] } df = pd.DataFrame(multi_data) row_quantiles = df.quantile(0.5, axis=1) print(row_quantiles)
This snippet calculates the median scores across each row (student in this scenario), demonstrating how the axis parameter can be used to either summarize rows or columns.
Handling Missing Data in Quantile Calculations
Adapt the
quantile()
function to manage datasets with missing values effectively.Employ parameters for handling nulls.
pythonincomplete_data = {'grades': [78, 90, None, 84]} df = pd.DataFrame(incomplete_data) quantile_with_na = df['grades'].quantile(0.5, interpolation='midpoint') print(quantile_with_na)
Given that one of the grade entries is
None
, specifying how Pandas handles such missing data through theinterpolation
parameter is crucial to gain accurate and sensible quantile values.
Conclusion
Mastering the quantile()
method in Pandas elevates your ability to analyze and understand the complexities of your data. By learning to compute quantiles in Pandas, extract significant statistical insights, and handle various kinds of data scenarios, you ensure robust data analysis and decision-making processes. Apply these techniques to improve the statistical grounding of your data manipulations, delivering clearer insights and more impactful data-driven strategies.
No comments yet.