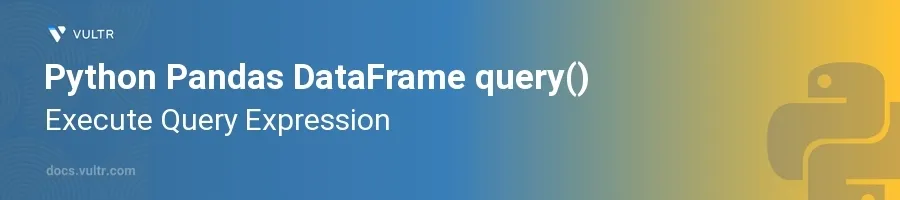
Introduction
The query()
method in Python's Pandas library offers a powerful way to perform query operations on DataFrame objects using a string expression. It is particularly useful for filtering data without directly using traditional Python logic, which can be verbose. This method enables concise and readable code, which can execute complex conditional queries on DataFrame columns.
In this article, you will learn how to leverage the query()
method to simplify data querying tasks in Pandas. The focus will be on creating and using query expressions to filter, analyze, and manipulate data in a DataFrame more efficiently than standard methods.
Basics of the query() Method
Understanding the Function Syntax
Recognize that
query()
allows for string expressions that reference column names directly.Know that the syntax closely resembles that used in SQL, making it intuitive for those familiar with SQL queries.
pythonimport pandas as pd # Create a simple DataFrame data = pd.DataFrame({ 'A': [1, 2, 3, 4, 5], 'B': [10, 20, 30, 40, 50], 'C': [100, 200, 300, 400, 500] }) # Querying data result = data.query('A < 4 and B > 15') print(result)
This code samples a DataFrame and uses
query()
to select rows where column 'A' is less than 4 and column 'B' is greater than 15. The result is a filtered DataFrame based on these conditions.
Evaluating Multiple Conditions
Combine multiple conditions within the query string using logical operators like
and
,or
.Use parentheses to manage precedence and clarify the query logic.
pythonresult = data.query('(A < 4 or A > 3) and B > 30') print(result)
In the example above, the expression filters rows where column 'A' is either less than 4 or greater than 3, and where column 'B' is greater than 30.
Advanced Usage of query()
Using Local Variables in Queries
Utilize the
@
symbol to reference local variables withinquery()
expressions.pythonmax_threshold = 30 result = data.query('B < @max_threshold') print(result)
In this code,
@max_threshold
is used to integrate a Python variable into the query, allowing for dynamic query adjustments based on external or computed values.
Handling String Operations
Use string methods within the query expression by accessing methods on string dtype columns using
str
.pythondata = pd.DataFrame({ 'Name': ['Alice', 'Bob', 'Charlie', 'David'], 'Age': [25, 30, 35, 40] }) result = data.query('Name.str.startswith("A") and Age > 20') print(result)
This snippet demonstrates filtering based on string operations, selecting names that start with 'A' and ages greater than 20.
Practical Applications of query() in Data Analysis
Filtering Data for Analysis
Build complex queries easily to filter dataframes based on business logic or analysis requirements.
Efficiently slice data without creating intermediate variables or complex filtering logic.
python# Example of more complex business logic data = pd.DataFrame({ 'Product': ['Widget', 'Gadget', 'Widget'], 'Sales': [200, 150, 300], 'Market': ['EU', 'US', 'EU'] }) result = data.query('Market == "EU" and Sales > 250') print(result)
Here, the
query()
method filters rows to show EU market products with sales more than 250 units. It demonstrates how effortlessly complex business logic can be implemented usingquery()
.
Conclusion
The query()
function in Pandas is a remarkably efficient and potent tool for manipulating and querying data within DataFrames. By using query expressions similar to those found in SQL, you can accomplish complex filtering and data analysis tasks with minimal code. Through practical examples, you now understand how to utilize local variables, string methods, and logical operators within your queries. Harness this function in your data analysis projects to keep your code clean and efficient while handling large sets of data.
No comments yet.