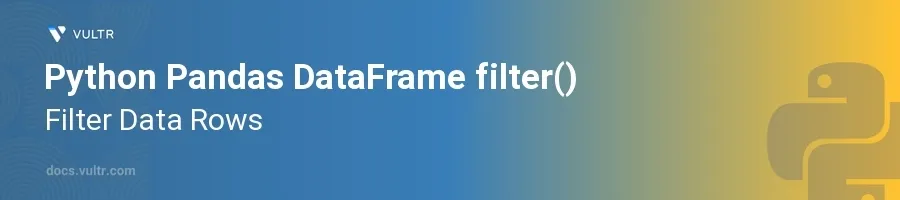
Introduction
The filter()
method in the Python Pandas library is an essential tool for refining datasets based on specific criteria in DataFrame rows or columns. This versatile function facilitates a focused analysis and manipulation of large datasets, allowing data scientists and analysts to extract necessary information quickly. Understanding how to effectively use the filter()
function is crucial for handling and cleaning data efficiently in Python.
In this article, you will learn how to adeptly use the filter()
function to filter rows in various scenarios with a Pandas DataFrame. Explore examples that demonstrate filtering data based on column names, based on conditions, dynamic filtration techniques, and filtering using regex.
Basics of Filtering DataFrame Rows Using filter()
Filtering DataFrame Using Column Names
Start by importing the Pandas library and creating a sample DataFrame for demonstration.
pythonimport pandas as pd data = {'Name': ['Tom', 'Nick', 'Krish', 'Jack'], 'Age': [20, 21, 19, 18], 'City': ['New York', 'London', 'San Francisco', 'Tokyo']} df = pd.DataFrame(data)
Apply
filter()
to select rows based on specific column names. Note thatfilter()
primarily filters columns by default, so using it directly for rows requires a different approach.
Filtering Based on Conditions
To filter rows based on conditions, consider combining
filter()
with boolean indexing or other functions likequery()
.pythonfiltered_df = df[df['Age'] > 19]
This code filters the DataFrame to only include rows where the age is greater than 19.
Understanding How Dynamic Filtering Works
Dynamic filtering involves adjusting your filtering criteria based on external variables or program logic.
Suppose you want your program to filter data based on an age provided by a user input:
pythonuser_age = 20 dynamic_filtered_df = df[df['Age'] > user_age]
This snippet takes a user-defined age and filters the DataFrame to include only rows where the age is greater than the specified value.
Advanced Techniques in Filtering Rows
Filtering Using Regular Expressions (Regex)
Using regex provides a powerful tool for complex filter conditions involving string patterns.
Suppose you want to filter all rows where the
City
column matches any city that begins with "San":pythonregex_filtered_df = df[df['City'].str.contains(r'^San')]
This code filters the DataFrame to only include rows where the city name starts with "San". The
^
in the regex pattern denotes the beginning of the string.
Combining Multiple Filters
Combine multiple conditions to refine your DataFrame selectively.
pythoncombined_filtered_df = df[(df['Age'] > 18) & (df['City'].str.contains(r'^New'))]
This example filters the DataFrame to include only rows where the age is greater than 18 and the city starts with "New".
Conclusion
Mastering the use of the filter()
function in Pandas greatly enhances your ability to manage and analyze data effectively. While filter()
is predominantly used to select specific DataFrame columns, combining it with techniques like boolean indexing, regex, and conditional filters allows for flexible and powerful row filtrations. Implement these filtration strategies to streamline your data analysis projects, ensuring you work only with the most relevant data for your analytical objectives. By leveraging these tips, make your data processing routines more efficient and targeted.
No comments yet.