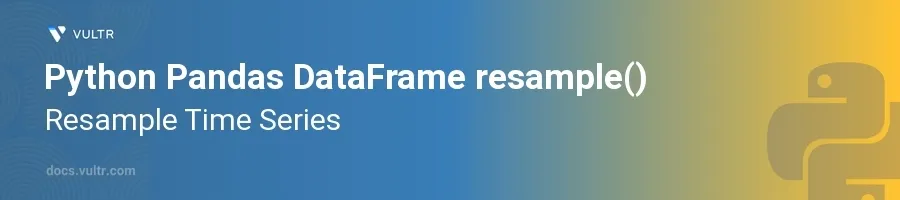
Introduction
The resample()
method in the Pandas library is a powerful tool for resampling time series data, allowing you to convert the time series to a specified frequency. This functionality is especially useful in financial analyses, weather data processing, and any field requiring time series manipulation to make data more digestible or to align it with other time series.
In this article, you will learn how to effectively utilize the resample()
method in various data manipulation scenarios involving time series. You'll explore practical examples that demonstrate how to downsample and upsample data, aggregate different time series data points, and utilize custom resampling strategies.
Basic Concepts of Resampling
Understanding the resample() Method
Import the Pandas library
Create a DateTime index using
pd.date_range()
Initialize a DataFrame with the DateTime index
Resample the data at a different frequency
pythonimport pandas as pd # Create a date range date_rng = pd.date_range(start='1/1/2022', end='1/10/2022', freq='D') # Create DataFrame df = pd.DataFrame(date_rng, columns=['date']) df['data'] = range(10) # Resample the DataFrame df_resampled = df.resample('2D', on='date').sum()
In this example, data is resampled from daily to a two-day frequency using
'2D'
. Thesum()
function aggregates values over each 2-day period.
Downsampling and Aggregating
Choose a downsampling frequency like 'W' for weekly
Apply an aggregation method like mean, sum, or custom function
pythonweekly_resampled = df.set_index('date').resample('W').mean()
This code changes the sampling frequency to weekly and calculates the average of data points within each week.
Advanced Resampling Techniques
Using Custom Resampling Functions
Define a custom function to customise data aggregation
Apply the custom function during the resampling process
pythondef custom_resample(array): return max(array) - min(array) df_custom_resampled = df.set_index('date').resample('3D').apply(custom_resample)
This approach uses a custom function that calculates the range (difference between max and min) over each period specified.
Resampling with Multiple Aggregations
Specify multiple aggregation functions simultaneously
Apply these aggregations to the resampled data
pythonresampled_multi_agg = df.set_index('date').resample('W').agg(['mean', 'sum', 'std'])
This example demonstrates how to compute the mean, sum, and standard deviation on a weekly basis.
Handling Missing Data in Resampling
Resample the original data set
Use methods like
fillna()
orinterpolate()
to handle missing data post-resamplingpythondaily_resampled = df.set_index('date').resample('D').mean().interpolate(method='linear')
This line demonstrates resampling to daily frequency, computing the mean, and using linear interpolation to fill in any resulting missing values.
Resampling Multi-Index DataFrames
Resampling When There are Multiple Levels in Index
Ensure the DateTime index is set with
set_index()
Apply resampling on a specific level using the
level
parameterpythonmulti_index_df = df.set_index(['category', 'date']) resampled_multi_index = multi_index_df.resample('M', level='date').sum()
Here, the resampling is applied at a monthly level on the date index of a multi-index DataFrame.
Conclusion
Mastering the resample()
method in Pandas allows you to manipulate time-series data effectively and flexibly. From basic downsampling and aggregation to complex scenarios involving multi-index DataFrames or custom aggregation functions, you've seen how versatile this tool can be in various analytical contexts. Apply these techniques to your datasets to improve the quality and interpretability of your temporal data analyses, ensuring more reliable and insightful outcomes.
No comments yet.