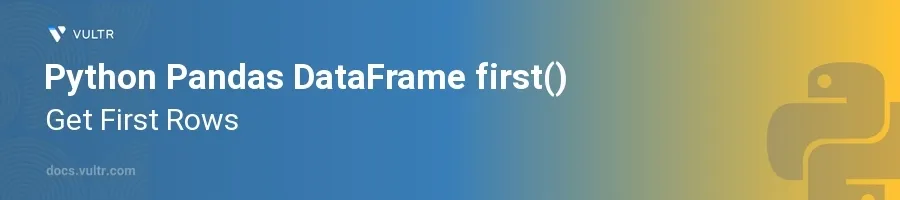
Introduction
The first()
method in Python's Pandas library is a powerful tool specifically designed for timestamp-indexed data. This method allows users to effortlessly extract the first few rows from a DataFrame based on a specified time period. It proves invaluable in analyzing time series data, where quick assessments of initial entries are frequently required.
In this article, you will learn how to utilize the first()
method effectively in various scenarios. Explore its functionality with different time intervals and understand how it can aid in simplifying data preprocessing and analysis tasks.
Understanding the first() Method
The first()
method is primarily used on DataFrame objects with datetime-like indexes. It enables the retrieval of the first rows based on a specific time delta from the start of the DataFrame. Here’s how to work with it:
Basic Usage of first()
Import Pandas and create a DataFrame with a datetime index.
Apply the
first()
method to obtain rows from the beginning of the DataFrame to a specified period.pythonimport pandas as pd import numpy as np # Creating a date range dates = pd.date_range('20230101', periods=6) # Creating a DataFrame df = pd.DataFrame(np.random.randn(6,4), index=dates, columns=list('ABCD')) print("Complete DataFrame:\n", df) print("\nFirst 3 days:\n", df.first('3D'))
This script creates a DataFrame
df
with dates as its index and appliesfirst('3D')
to fetch the rows from the first three days of the index.
Specifying Different Time Periods
Realize that the
first()
method accepts various time specifications like days (D
), minutes (T
), and seconds (S
).Use this flexibility to tailor data extraction based on the datetime precision required.
python# Example using hours print("First 5 hours:\n", df.first('5H'))
In this example, by specifying
'5H'
, the method returns the rows from the first five hours from the start of the DataFrame index.
Practical Applications
The first()
method can significantly enhance data analysis efficiency. Here are some practical ways to leverage this method across different scenarios:
Analyzing Financial Data
For financial datasets with timestamps, quickly summarize initial market activities.
Use the
first()
method in combination with other Pandas functions to perform initial trend analysis.python# Suppose df is a DataFrame of stock prices with a datetime index print("Initial trading period analysis:\n", df.first('30T').describe())
Here, summarizing the first 30 minutes of trading can provide insights into the opening market behavior.
Event-Driven Data Analysis
When dealing with event logs that have timestamped entries, utilize
first()
to inspect the commencement of events or processes.Combine it with filtering techniques to focus on specific event types or categories within the initial time frame.
python# Assuming df contains event data with types initial_events = df[df['EventType'] == 'Login'].first('15T') print("First 15 minutes of login events:\n", initial_events)
This snippet filters the DataFrame to only 'Login' events and then applies
first('15T')
to analyze the early phase of logins.
Conclusion
The first()
function in Pandas is a versatile and robust tool for handling time-indexed data efficiently. By allowing precise control over the time range, it facilitates quick data extraction and initial analysis, which is essential in many real-time data applications and studies. Tailor its use to fit specific time intervals to enhance the granularity of analysis and make informed decisions based on the early data trends in your DataFrame. By integrating these strategies, streamline your data processing workflows effectively.
No comments yet.