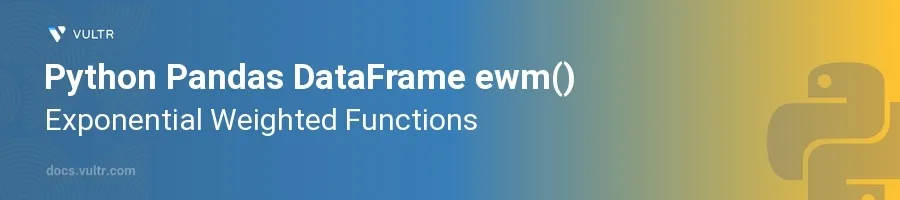
Introduction
The ewm()
function is an integral method in Python’s Pandas library, particularly when dealing with time series data. This method provides functionalities to compute Exponential Moving Averages (EMA) or other exponentially weighted statistics over a specified window. EMA is particularly useful in financial analysis and economic forecasting because it prioritizes more recent data points, thus reacting more significantly to recent changes in data compared to simple moving averages (SMA).
In this article, you will learn how to effectively utilize the ewm()
function to calculate exponential moving averages and other related statistics. Explore robust examples and applications of this function in handling real world data sets, and grasp how to implement and customize exponential weighting for diverse analytical needs.
Understanding ewm() in Pandas DataFrame
Basic Configuration of ewm()
Import the Pandas library and create a sample DataFrame.
Configure the
ewm()
function with basic parameters likespan
oralpha
.pythonimport pandas as pd import numpy as np # Create a DataFrame data = np.random.randn(10) df = pd.DataFrame(data, columns=['random']) # Apply ewm ewm_df = df['random'].ewm(span=3, adjust=False).mean() print(ewm_df)
In this example, a DataFrame containing random data points is created, and the
ewm()
method is applied to the column "random". The parameterspan=3
defines the decay in terms of span for the EMA calculation. Theadjust=False
param ensures that the weighted averages are calculated with equal weights.
Span vs Halflife vs Alpha
Understand that
span
,halflife
, andalpha
are parameters that define the decay rate for the exponential weighting.Use each in different settings to control the rate according to your data sensitivity need.
python# Using halflife ewm_halflife = df['random'].ewm(halflife=2, adjust=True).mean() # Using alpha directly ewm_alpha = df['random'].ewm(alpha=0.1, adjust=True).mean() print("EWMA using halflife:\n", ewm_halflife) print("EWMA using alpha:\n", ewm_alpha)
The
halflife
parameter defines the period it takes for the weight to reduce by half, whilealpha
explicitly sets the smoothing factor. Adjusting these parameters helps tailor the sensitivity of the EMA to your specific data trends.
Advanced Usage of ewm()
Applying ewm() to Multiple Columns
Create a DataFrame with multiple data columns.
Apply exponential weighting to each column using
ewm()
.pythonmulti_data = pd.DataFrame(np.random.randn(10, 3), columns=['A', 'B', 'C']) ewm_multi = multi_data.ewm(span=3).mean() print(ewm_multi)
This allows performing EMA across multiple columns, useful for concurrent analysis of correlated data streams in applications like multivariate time-series forecasting.
Using ewm() with Custom Functions
Use
apply()
along withewm()
to incorporate custom functions for more complex statistics.pythoncustom_ewm = df['random'].ewm(span=3).apply(lambda x: np.sum(x**2)) print(custom_ewm)
The lambda function in the apply method computes the sum of squares of the data, weighed exponentially. This is particularly useful if you need to analyze variance or other higher-order statistics with exponential weighting.
Visualizing Exponential Weighted Data
Utilize visualization libraries such as Matplotlib to chart EMA analyses.
Compare exponential weighted data with original data.
pythonimport matplotlib.pyplot as plt plt.plot(df.index, df['random'], label='Original') plt.plot(ewm_df.index, ewm_df, label='Exponential Weight', linestyle='--') plt.legend() plt.show()
This step helps in visually contrasting the original data against the exponentially smoothed data, illuminating trends and anomalies more clearly.
Conclusion
EWM functions like ewm()
in Pandas offer valuable flexibility for smoothing data series, adapting to changes in data more dynamically than simple averages. By understanding and applying different configurations of the ewm()
function, you enhance data analysis workflows with robust, sensitive handling of fluctuations in sets from stock prices to IoT sensor streams. Harness these examples to refine your time-series data exploration and uncover insights with precision and efficiency.
No comments yet.