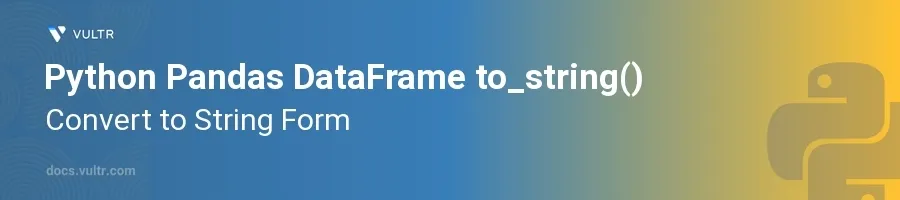
Introduction
Pandas, a powerful data manipulation library in Python, provides numerous functions for effective data analysis and transformation. One such function is to_string()
, which converts a DataFrame into its string representation. This function is particularly useful when you need to display or log the DataFrame in a text format for reporting, debugging, or other similar tasks.
In this article, you will learn how to utilize the to_string()
method in various contexts to convert a Pandas DataFrame into a string. You'll explore different parameters that customize the string output according to various requirements, such as controlling the number of displayed rows and columns, as well as modifying header and index visibility.
Basics of to_string()
Converting an Entire DataFrame to a String
Begin with a basic example of converting a complete DataFrame to a string.
pythonimport pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]} df = pd.DataFrame(data) print(df.to_string())
Here, a DataFrame is created using a dictionary with names and ages. The
to_string()
method converts the entire DataFrame into a string format and prints it, displaying all rows and columns.
Handling Large DataFrames
Understand the behavior when dealing with larger DataFrames.
When dealing with a large DataFrame that contains many rows or columns, Pandas might truncate the string representation to fit the output neatly into your console. The
to_string()
function provides parameters to manage this behavior.Utilize the
max_rows
andmax_cols
parameters to control output.pythonimport numpy as np # Create a large DataFrame large_data = pd.DataFrame(np.random.rand(100, 10)) # Convert to string with limited rows and columns displayed string_representation = large_data.to_string(max_rows=10, max_cols=5) print(string_representation)
This code snippet generates a large DataFrame with random numbers and limits the output to the first and last five rows and the first five columns.
Customizing String Output
Adjusting Index and Header Visibilities
Adjust the visibility of the headers and the index in the output string.
The
to_string()
method allows controlling whether to include headers and the index using theindex
andheader
parameters.pythondf = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) # Convert DataFrame to string without index and header print(df.to_string(index=False, header=False))
In the above example, converting the DataFrame to string excludes both the index and the header, resulting in a simple list of values.
Formatting Floating Point Numbers
Customize the format of floating-point numbers using the
float_format
parameter.pythondf = pd.DataFrame({'Data': [3.14159, 2.71828, 1.41421]}) # Formatting with two decimal places print(df.to_string(float_format='{:.2f}'.format))
This example demonstrates how to format floating-point numbers to display them with two decimal places in the string output.
Including or Excluding Specific Columns
Use the
columns
parameter to specify which columns to include in the output.pythondata = {'Name': ['Alice', 'Bob'], 'Age': [30, 25], 'Occupation': ['Engineer', 'Doctor']} df = pd.DataFrame(data) # Display only Name and Occupation columns print(df.to_string(columns=['Name', 'Occupation']))
Here, the
to_string()
method is used to generate a string that includes only the 'Name' and 'Occupation' columns, ignoring the 'Age' column.
Conclusion
The to_string()
function in Pandas offers a robust way to convert a DataFrame to its string representation, making it easier to view or log the DataFrame in a text format. By exploring various parameters such as max_rows
, max_cols
, index
, header
, and float_format
, optimize the string output to meet specific needs. Whether it’s handling large datasets, customizing numerical formats, or selectively displaying data, to_string()
enhances the flexibility and readability of your data representation tasks in Python. Use these techniques to ensure your data outputs are precise and tailored for any situation.
No comments yet.