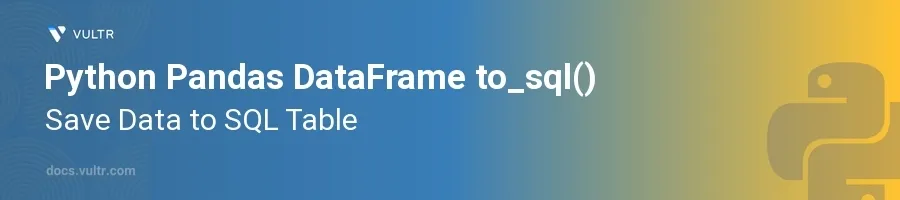
Introduction
The to_sql()
function from the pandas library in Python offers a straightforward way to write DataFrame data to an SQL database. This function is crucial for data scientists and developers who need to interact with databases seamlessly within their Python code. Whether you're logging data, updating your database, or integrating Python scripts with SQL database operations, to_sql()
helps make these tasks efficient and error-free.
In this article, you will learn how to utilize the to_sql()
function to save pandas DataFrames to an SQL table. Explore how to set up a DataFrame, connect to a database using SQLAlchemy, and write the DataFrame to an SQL table while managing different parameters like table schema, data insertion method, and handling index labels.
Understanding DataFrame to SQL Table Conversion
Preparing Your DataFrame
Choose or create a DataFrame to work with. Consider a dataset that represents daily sales records.
pythonimport pandas as pd data = {'Date': ['2021-01-01', '2021-01-02', '2021-01-03'], 'Product_ID': [101, 102, 103], 'Sold_Units': [29, 19, 25]} df = pd.DataFrame(data)
This DataFrame
df
contains sales records with columns for the date, product ID, and the units sold.
Setting Up Database Connection
Use SQLAlchemy to create a connection to your SQL database. Assume a SQLite database in this example.
pythonfrom sqlalchemy import create_engine engine = create_engine('sqlite:///my_sales_data.db')
The connection to the database is established using SQLAlchemy's
create_engine()
, specifying SQLite as the database system.
Writing DataFrame to SQL Table Using to_sql()
Write the DataFrame into an SQL table with the
to_sql()
function. Handle the table name, the SQLAlchemy engine, and additional parameters like the if_exists argument.pythondf.to_sql('daily_sales', con=engine, if_exists='replace', index=False)
Here,
to_sql()
saves the DataFramedf
to an SQL table nameddaily_sales
. Theif_exists='replace'
parameter ensures the table is dropped and created anew if it exists. Settingindex=False
does not write the DataFrame index as a separate column in the table.
Customizing the to_sql() Function
Including Indexes
Save the DataFrame such that the DataFrame index is also saved in the SQL table.
pythondf.index = pd.Index([1, 2, 3]) # Setting a specific index df.to_sql('daily_sales', con=engine, if_exists='append', index=True, index_label='ID')
This command saves the DataFrame to the SQL table with the index preserved under the column name 'ID'.
Handling Data Types
Control the types of the columns which are saved into the SQL table by specifying them explicitly.
pythondf.to_sql('daily_sales', con=engine, if_exists='append', dtype={'Date': sqlalchemy.types.DATE, 'Product_ID': sqlalchemy.types.Integer(), 'Sold_Units': sqlalchemy.types.Integer()})
Specifying the column data types directly in the
to_sql()
function helps ensure compatibility with SQL data types, avoiding common pitfalls like type mismatches.
Conclusion
The to_sql()
function in pandas is an essential tool for developers and analysts dealing with data interplay between Python and SQL databases. It simplifies transferring data directly from a DataFrame into an SQL table, accounting for various intricacies like data types, indexes, and database schema updates. By deploying this function, streamline database management tasks, and maintain data integrity between your applications and storage solutions. With the techniques covered, take advantage of this powerful function to enhance data operations within your projects.
No comments yet.