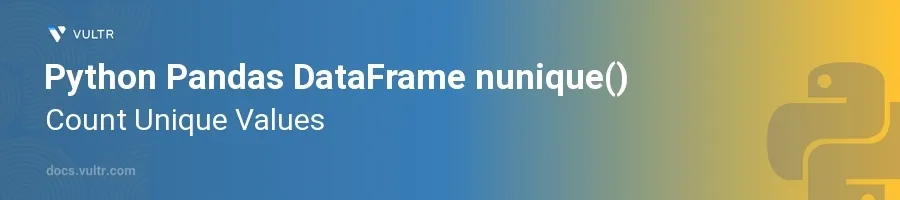
Introduction
The nunique()
method in the Python Pandas library is an essential tool for data analysis, particularly when needing to count unique values within a DataFrame column. This function simplifies the task of determining the diversity or variability of data, which is crucial for tasks like data cleaning, preprocessing, and understanding data distributions.
In this article, you will learn how to effectively leverage the nunique()
method across various data scenarios in Pandas. Dive into practical examples that demonstrate counting unique values in entire DataFrames, specific columns, and even considering missing values properly.
Understanding nunique() in Pandas
The nunique()
method returns the number of unique elements in the object. It implicitly ignores NaN
values by default, providing an accurate count of actual data points. Explore its functionality through detailed examples.
Count Unique Values in a Single Column
Import the Pandas library and create a DataFrame with sample data.
Apply the
nunique()
method to a specific column to count unique values.pythonimport pandas as pd data = {'Name': ['Alice', 'Bob', 'Alice', 'David', 'Bob'], 'Age': [25, 30, 25, 40, 30]} df = pd.DataFrame(data) unique_names = df['Name'].nunique() print("Number of unique names:", unique_names)
Here, the DataFrame
df
includes repeated names. By usingdf['Name'].nunique()
, obtain the count of unique names, which in this case is 3: Alice, Bob, and David.
Count Unique Values in the Entire DataFrame
Utilize the
nunique()
method on the entire DataFrame.Use the
axis
parameter to count across different dimensions.pythonunique_per_column = df.nunique() print("Unique values per column:\n", unique_per_column) unique_per_row = df.nunique(axis=1) print("\nUnique values per row:\n", unique_per_row)
This example first counts unique entries in each column and then counts unique values per row by specifying
axis=1
. This way, evaluate uniqueness both vertically and horizontally, useful in data summary and anomaly detection.
Considering NaN values in Unique Counts
Include
NaN
values in a DataFrame to see the default behavior.Set
dropna=False
to includeNaN
values in the count.pythondata_with_missing = {'Color': ['Red', 'Blue', 'Red', 'Green', None]} df_missing = pd.DataFrame(data_with_missing) unique_colors = df_missing['Color'].nunique() # Default dropna=True print("Unique colors (excluding NaN):", unique_colors) unique_colors_incl_nan = df_missing['Color'].nunique(dropna=False) print("Unique colors (including NaN):", unique_colors_incl_nan)
By executing this code with and without the
dropna
parameter, see howNaN
is treated as a unique value whendropna
is set toFalse
. This adjustment is essential when all data, including missing or undefined values, counts towards the analysis.
Advanced Scenarios and Best Practices
Encounter more complex situations as data grows in intricacy and size. Here are extended considerations for using nunique()
:
- Combining with other Pandas functions: Integrate
nunique()
with functions likegroupby()
to count unique values within subgroups of your data. - Performance considerations: On very large datasets, keep in mind that counting unique values can be computationally expensive. Optimize by filtering data or sampling where appropriate.
- Data exploration: Use
nunique()
during exploratory data analysis to get a sense of data cardinality and distribution, which can inform feature engineering and model training.
Conclusion
Pandas' nunique()
method is a powerful function for counting unique values in a DataFrame, helping to glean insights into the diversity and distribution of data. Employ nunique()
to ensure data is comprehensively understood, whether confirming data integrity, preparing data for machine learning, or conducting exploratory data analysis. By mastering its usage through the examples and best practices provided, ensure analysis is informed, precise, and efficient.
No comments yet.