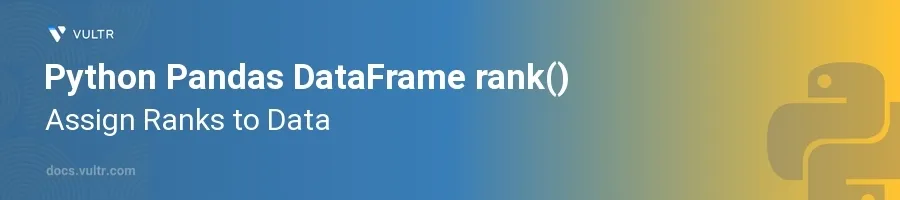
Introduction
Ranking data is a fundamental task in data analysis, especially when you need to compare elements, prioritize items, or handle tie-breakers in datasets. Python's Pandas library simplifies ranking tasks with the rank()
method for DataFrame objects. This method provides extensive flexibility through its various parameters, allowing fine control over how rankings are computed and displayed.
In this article, you will learn how to effectively utilize the rank()
method provided by Pandas DataFrame to assign ranks. Discover how to rank numerical and categorical data, handle ties with different strategies, and explore variations in ranking such as ascending or descending order.
Understanding the rank() Method
Basic Usage of rank()
Import the Pandas library and create a DataFrame.
Apply the
rank()
method to assign ranks to data in the DataFrame.pythonimport pandas as pd data = {'Score': [250, 400, 300, 300, 150]} df = pd.DataFrame(data) df['Rank'] = df['Score'].rank() print(df)
This code snippet creates a DataFrame with scores and uses the
rank()
method to assign ranks. Note that by default,rank()
deals with ties by assigning each tied value the average rank.
Rank Handling of Ties
Explore different methods using the
method
parameter inrank()
to handle ties explicitly.Apply methods like 'average', 'min', 'max', 'first', and 'dense' to see how each treats ties.
pythondf['Rank_min'] = df['Score'].rank(method='min') df['Rank_max'] = df['Score'].rank(method='max') df['Rank_first'] = df['Score'].rank(method='first') df['Rank_dense'] = df['Score'].rank(method='dense') print(df)
Each ranking method treats ties differently: 'min' assigns the lowest rank in the group, 'max' gives the highest, 'first' considers the order in the data, and 'dense' compresses ranks without gaps.
Advanced Ranking Techniques
Assigning Ranks in Descending Order
Use the
ascending=False
parameter inrank()
to order ranks in descending order.Re-run the ranking after modifying the order for a reverse interpretation of importance.
pythondf['Rank_descending'] = df['Score'].rank(ascending=False) print(df)
Ranking in descending order typically places the highest value with the highest rank, reversing the default behavior where the lowest value gets the lowest rank.
Ranking Different Data Types
Extend the ranking concept to other data types like timestamps or categorical data.
Convert categorical data or timestamps into sortable types if necessary and then rank.
pythondf['Date'] = pd.to_datetime(['2022-01-01', '2022-01-02', '2022-01-02', '2022-01-03', '2022-01-04']) df['Date_rank'] = df['Date'].rank() print(df[['Date', 'Date_rank']])
The
rank()
method can also be applied to dates and times. Here, the method assigns ranks based on the chronological order of dates.
Custom Ranks with Pct Parameter
Using the pct=True Parameter
Set
pct=True
in therank()
method to get the relative ranking as a percentage.This approach normalizes the ranking results between 0 and 1, which is useful for cross-analysis.
pythondf['Rank_pct'] = df['Score'].rank(pct=True) print(df[['Score', 'Rank_pct']])
When
pct=True
is used, the ranks are expressed as a percentage of the total count, offering a direct comparison of an individual score's position relative to the dataset.
Conclusion
The rank()
function in Pandas is a potent tool for assigning ranks and handling comparisons within data sets. You master handling numerical, categorical, or even date-focused data ranking, and address tie strategies comprehensively. This functionality boosts data analysis, especially when prioritizing or grouping elements based on their values or other specific criteria. By adopting these techniques, you ensure more effective data management and clearer analytical outcomes in your Python projects.
No comments yet.