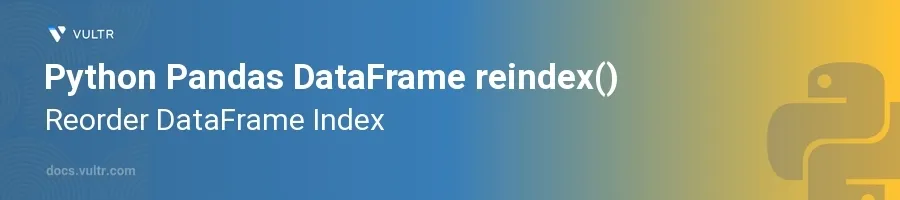
Introduction
The reindex()
method in Python's Pandas library is a powerful tool for modifying the row or column labels of a DataFrame. This function allows you to conform a dataset to a new set of labels along a specific axis, handling nonexistent labels appropriately either by inserting missing values (NaNs) or by filling them using a method like forward fill or backward fill. reindex()
is crucial for data alignment and ensuring consistent structure, especially when working with time-series data or when combining datasets that don’t initially align.
In this article, you will learn how to effectively use the reindex()
method to reorder indices in a DataFrame. Explore how to customize the method for various scenarios, including reordering rows and columns, using different filling strategies for missing indices, and managing non-unique labels.
The Basics of Reindexing
Reordering DataFrame Rows
Reindexing isn’t just about changing the order of index labels; it’s about aligning data to a new label set. Here’s how you can achieve this:
Import Pandas and create a sample DataFrame.
pythonimport pandas as pd data = {'Name': ['John', 'Anna', 'James', 'Linda'], 'Age': [28, 22, 35, 32]} df = pd.DataFrame(data)
Define a new index order and use
reindex()
to rearrange the rows.pythonnew_order = [3, 0, 2, 1] df_reindexed = df.reindex(new_order) print(df_reindexed)
After running the code, the DataFrame
df
will reflect the new order specified bynew_order
, where the rows are rearranged according to the new indices.
Handling Missing Indices
In scenarios where the new index contains elements not present in the original DataFrame, reindex()
can handle these gracefully:
Specify a new index that includes nonexistent labels.
pythonextended_index = [0, 1, 2, 3, 4, 5] # Note that original DataFrame has indices 0, 1, 2, 3 only df_extended = df.reindex(extended_index) print(df_extended)
You will notice that rows corresponding to indices 4 and 5 are filled with NaN because these indices do not exist in the original DataFrame.
Using Fill Methods
reindex()
also allows you to specify how to handle filling missing data:
Use the
method
parameter to auto-fill missing values. Common methods include 'ffill' for forward fill and 'bfill' for backward fill.pythondf_filled = df.reindex(extended_index, method='ffill') print(df_filled)
This code snippet demonstrates how 'ffill' (forward fill) propagates the last valid observation forward. Hence, missing indices will be filled with the last available values.
Reindexing DataFrame Columns
Just like rows, columns can also be reindexed to change their order or introduce new columns.
Reordering Columns
Depending on your analysis needs, reordering columns may be necessary to better organize data.
Create a DataFrame and reorder its columns using
reindex()
.pythoncolumns_reordered = ['Age', 'Name'] df_columns_reordered = df.reindex(columns=columns_reordered) print(df_columns_reordered)
In this example, the columns have been swapped to place 'Age' before 'Name', altering the default presentation.
Introducing New Columns
You can introduce new columns and specify how to handle their absence.
Include new columns in the reindexing process.
pythonnew_columns = ['Name', 'Age', 'Gender'] df_new_columns = df.reindex(columns=new_columns) print(df_new_columns)
Here, a new column 'Gender' is added, filled with NaN since no initial data exists for this column in the provided DataFrame.
Conclusion
The reindex()
function in Pandas is an essential tool for realigning data to a new set of labels. Whether you’re adjusting the order of rows and columns, handling missing data with intelligent fill methods, or adding new dimensions to your analytical framework, reindex()
offers a flexible solution. With the practical approaches outlined, optimize the structure of your DataFrames to meet the rigorous demands of data analysis, ensuring that your data is clean, organized, and ready for any challenge ahead.
No comments yet.