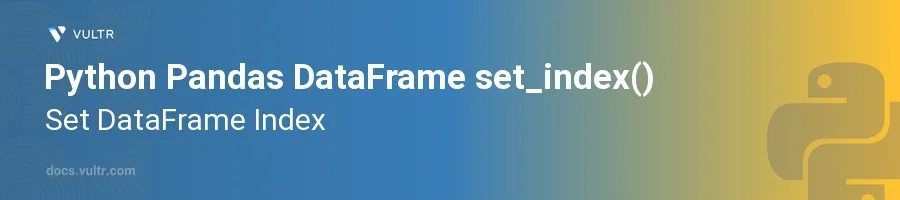
Introduction
Pandas is a powerful library in Python widely used for data manipulation and analysis, particularly through its prominent DataFrame structure. Among the numerous functionalities provided by the DataFrame, one key method is set_index()
. This method is crucial when you need to set a specific column as the index of the DataFrame, which can be pivotal for data slicing, dicing, and more efficient retrievals.
In this article, you will learn how to harness the set_index()
function on DataFrame objects in Pandas. This tutorial offers guidance on setting indices with single or multiple columns, resetting the index, and the nuances that come with each approach in data analysis.
Understanding set_index()
The set_index()
function in Pandas is used primarily for setting a column or multiple columns as the new index of the DataFrame. One of the main perks of setting a specific column as an index is the increased efficiency in data retrieval operations. It can also help in performing joins and merges more seamlessly by having indices on which these operations are naturally optimized.
Set Index Using a Single Column
Start with importing Pandas and creating a sample DataFrame.
Choose a column which you want to set as the new index.
Utilize the
set_index()
function to modify the index.pythonimport pandas as pd data = { 'Product ID': [1001, 1002, 1003, 1004], 'Product Name': ['WidgetA', 'WidgetB', 'WidgetC', 'WidgetD'], 'Price': [12.50, 15.50, 8.75, 9.50] } df = pd.DataFrame(data) df = df.set_index('Product ID') print(df)
Here, setting 'Product ID' as the index makes it the new row identifier replacing the default integer index.
Set Multiple Columns as Index
Recognize scenarios where a combination of multiple columns serves as a better index.
Choose the appropriate columns and use
set_index()
accordingly.pythondf = pd.DataFrame(data) df = df.set_index(['Product ID', 'Price']) print(df)
Using multiple columns as an index can be useful for hierarchical indexing, which plays an important role in various multi-level data arrangements.
Using inplace=True
to Avoid Copy
Understand that
set_index()
by default returns a new DataFrame unless specified otherwise.Use the
inplace=True
flag to modify the DataFrame in place.pythondf.set_index('Product ID', inplace=True)
Setting
inplace=True
modifies the original DataFrame directly, conserving memory and processing time by avoiding the creation of a new DataFrame object.
Resetting the Index
After setting a new index, you might need to revert to a default index or rearrange the indices. This is where reset_index()
comes in.
Reset to Default Integer Index
Use the
reset_index()
function to revert your DataFrame to the default numerical index.pythondf.reset_index(inplace=True)
This restores the DataFrame to its original form, with a default integer index and the previously set index turning back into a regular column.
Dropping the Index Column on Reset
Decide whether you want to drop the column used as an index entirely when resetting.
Employ the
drop=True
parameter if the old index is no longer needed.pythondf.reset_index(drop=True, inplace=True)
This approach is useful when the index column is no longer required, ensuring cleaner and more relevant DataFrame structure for further data operations.
Conclusion
The set_index()
function in Pandas provides a versatile tool to manipulate DataFrame indices efficiently, whether setting single or multiple columns as indices. Mastering this function enriches your data handling capabilities in Python, allowing for more adept data manipulation, efficient retrieval, and optimum use of the DataFrame structure. By diving into these techniques, you enhance your data analysis skills through adept handling of indices in Pandas DataFrames.
No comments yet.