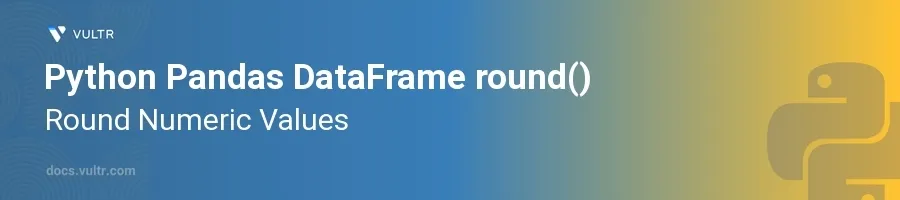
Introduction
The round()
function in pandas is a crucial tool for managing data precision across numerical datasets, often essential when dealing with large data frames or preparing data for presentation. It helps ensure consistency and clarity by modifying the floating-point values to a specified number of decimal places. Operating on DataFrame objects, this function makes it straightforward to round off all or selected numerical columns, enhancing data interpretability.
In this article, you will learn how to efficiently utilize the round()
function to adjust the numerical accuracy of DataFrames in the Python pandas library. Get to grips with various techniques to apply rounding on whole data frames or specific columns, and understand how to control rounding behavior through function parameters.
Understanding the round() Function
Basic Usage of round()
Import the pandas library and create a DataFrame with floating-point numbers.
Apply the
round()
function to the entire DataFrame.pythonimport pandas as pd data = {'A': [2.333, 5.659, 1.123], 'B': [0.564, 0.9999, 2.365]} df = pd.DataFrame(data) # Rounding all data to 1 decimal place rounded_df = df.round(1) print(rounded_df)
This block of code first creates a DataFrame from a dictionary of lists, each containing floating-point numbers. The DataFrame is then rounded off to one decimal place across all numeric columns using the
round()
function. The output reflects the rounded values.
Selective Column Rounding
Define the number of decimal places for each column individually using a dictionary.
Pass the dictionary to the
round()
function to apply column-specific rounding.python# Dictionary specifying rounding per column rounding_dict = {'A': 2, 'B': 0} # Applying selective rounding selectively_rounded_df = df.round(rounding_dict) print(selectively_rounded_df)
This snippet demonstrates how to selectively round each column by providing a dictionary where keys are column names and values are the decimal places to which to round. Column 'A' rounds to two decimal places, while Column 'B' rounds to zero, effectively making it integer.
Addressing Floating Point Precision Issues
Rounding and Data Analysis
Understand that rounding can affect summarizations like mean, sum, and more.
Use rounding judiciously to maintain data integrity during analytical operations.
python# Calculating sum before and after rounding sum_before = df['A'].sum() sum_after = rounded_df['A'].sum() print("Sum before rounding:", sum_before) print("Sum after rounding:", sum_after)
This example calculates the sum of column 'A' before and after rounding to illustrate how numerical operations might differ due to rounding. Such discrepancies can be significant depending on the data and the required precision for analysis or reporting.
Fine-Tuning Rounding with numpy
Integrate NumPy for more complex rounding rules like rounding towards zero, ceil, or floor.
Employ the combination of pandas and NumPy to manage rounding tasks where default rounding isn't sufficient.
pythonimport numpy as np # Using NumPy to round down towards zero df['A'] = np.floor(df['A']) print(df)
This code uses NumPy's
floor()
function to forcefully round down all values in column 'A', which can be useful in scenarios where such a rounding strategy is warranted (e.g., during inventory or stock calculations).
Conclusion
Mastering the round()
function in pandas enriches your data manipulation toolkit, enabling you to refine how numerical data is displayed and used, particularly in data processing pipelines or analytical reporting. By adjusting the precision of DataFrame columns, you ensure that data is meaningful and fit for purpose, whether for detailed technical analysis or executive summaries. Utilize this function with an understanding of both its capabilities and its impact on data interpretation, ensuring that the precision needed matches the context of your work.
No comments yet.