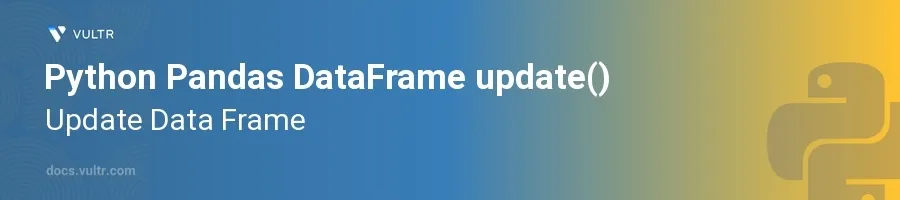
Introduction
The update()
function in Pandas is a powerful tool designed to modify a DataFrame in-place using values from another DataFrame. This function is particularly useful for updating a dataset without the need for complex loops or applying conditions explicitly, streamlining the process of ensuring data consistency and integrity across DataFrame objects.
In this article, you will learn how to utilize the update()
function effectively to amend data within your DataFrames. You are introduced to practical examples illustrating how to apply this method for different use cases like updating with and without overriding existing data, and handling missing values or different data types.
Understanding the update() Method
Basic Usage of update()
Consider two DataFrames, where one serves as the original dataset and the other contains updated values you wish to merge.
Use the
update()
method to modify the original DataFrame based on non-NA values in the second DataFrame.pythonimport pandas as pd df_original = pd.DataFrame({'A': [1, 2, 3], 'B': [400, 500, 600]}) df_new = pd.DataFrame({'A': [4, pd.NA, 6], 'B': [700, 800, 900]}) df_original.update(df_new) print(df_original)
This code updates
df_original
using values fromdf_new
where they are not NA. The output reflects changes in both columns A and B, except in places wheredf_new
contains NA values.
Overriding vs. Non-overriding Updates
Decide whether to override the original data if the updated data has missing values.
Apply the
update()
function with the appropriate parameters to achieve the desired outcome.pythondf_original = pd.DataFrame({'A': [1, 2, 3], 'B': [400, None, 600]}) df_update = pd.DataFrame({'A': [None, None, 5], 'B': [700, 800, None]}) df_original.update(df_update, overwrite=False) print(df_original)
In this example, setting
overwrite=False
prevents the original DataFrame from updating where the new data is missing (None). Consequently, the original values remain intact whereverdf_update
has None.
Advanced Usage of update()
Handling Different Data Types
Understand how
update()
deals with different data types in the two DataFrames.Ensure data types match between the original and the updating DataFrame to prevent unintended data type conversion.
pythondf_original = pd.DataFrame({'A': [1.0, 2.0, 3.0], 'B': ['x', 'y', 'z']}) df_update = pd.DataFrame({'A': [4, pd.NA, 6], 'B': [None, 'w', 'u']}) df_original.update(df_update) print(df_original)
This code snippet shows an update where numerical and string data types are correctly managed, adhering to type integrity across the DataFrame.
Utilize Filtering for Conditional Updates
Create conditions to filter which parts of the DataFrame are to be updated.
Combine filtering techniques with the
update()
method to selectively update data.pythondf_original = pd.DataFrame({'A': [1, 2, 3], 'B': [10, 20, 30]}) df_conditional_update = pd.DataFrame({'A': [100, None, 300], 'B': [None, 200, 300]}) condition = df_conditional_update['A'] > 50 df_filtered = df_conditional_update[condition] df_original.update(df_filtered) print(df_original)
The update only happens for rows where column 'A' values in
df_conditional_update
are greater than 50. This demonstrates the conditional application of updates, preserving the original data where conditions aren't met.
Conclusion
The update()
method in Pandas simplifies the process of synchronizing changes between DataFrame objects. Whether dealing with overriding data, handling different data types, or applying conditional updates, this function helps maintain data integrity and unity efficiently. Use it to ensure that your data remains consistent and up-to-date without complex manipulations. Remember, mastering the use of update()
significantly enhances the manageability of DataFrame operations within Python.
No comments yet.