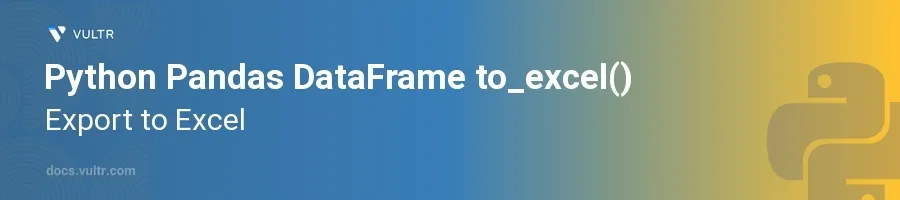
Introduction
Pandas is a powerful Python library for data manipulation and analysis, particularly suited for working with structured data such as tables, series, and matrices. A common task in data analysis involves exporting data to different formats for presentation, sharing, or further processing. Among these formats, Excel files are widely used due to their accessibility and ubiquity in business environments.
In this article, you will learn how to utilize the to_excel()
method in Pandas to export DataFrame objects to Excel files. Explore different customization options available with to_excel()
, such as specifying sheet names, deciding which columns to export, and handling different datatypes seamlessly.
Understanding the to_excel() Method
The to_excel()
function in Pandas allows for exporting a DataFrame to an Excel file, offering various parameters to customize the export process. Before diving into specific examples and customization, it's essential to grasp the basic usage of this method.
Basic Usage of to_excel()
First, ensure that Pandas is installed in your Python environment.
Import Pandas and create a simple DataFrame.
Use
to_excel()
to export the DataFrame to an Excel file.pythonimport pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'Occupation': ['Engineer', 'Doctor', 'Artist']} df = pd.DataFrame(data) df.to_excel('output.xlsx')
This code snippet creates a DataFrame from a dictionary, then exports it to an Excel file named
output.xlsx
. The file will appear in the current working directory unless a specific path is provided.
Specifying Sheet Names
Create a DataFrame as previously shown.
Use the
sheet_name
parameter to specify a custom name for the sheet in the Excel file.pythondf.to_excel('output.xlsx', sheet_name='Employees')
Here, the exported Excel file will contain a single sheet named 'Employees', where the DataFrame data is written.
Exporting Specific Columns
Define a DataFrame with multiple columns.
Specify columns to export using the
columns
parameter.pythondf.to_excel('output_custom_columns.xlsx', columns=['Name', 'Occupation'])
This will export only the 'Name' and 'Occupation' columns to the Excel file, excluding the 'Age' column.
Exporting Multiple DataFrames to a Single Excel File
It is often useful to export multiple DataFrames to a single Excel file, each in a separate sheet. This can be accomplished using the ExcelWriter
object provided by Pandas.
Using ExcelWriter to Manage Multiple Sheets
Import
ExcelWriter
from Pandas.Create multiple DataFrames.
Use
ExcelWriter
to export these DataFrames to multiple sheets within a single Excel file.pythonimport pandas as pd from pandas import ExcelWriter df1 = pd.DataFrame({'Data': [1, 2, 3]}) df2 = pd.DataFrame({'Data': [4, 5, 6]}) with ExcelWriter('multiple_sheets.xlsx') as writer: df1.to_excel(writer, sheet_name='First') df2.to_excel(writer, sheet_name='Second')
This example uses
ExcelWriter
to create an Excel file that contains two sheets, 'First' and 'Second', with data fromdf1
anddf2
, respectively.
Conclusion
Exporting data to Excel using the to_excel()
method in Pandas provides a straightforward yet flexible way to handle data sharing and reporting in Python. By utilizing the customization options available, such as specifying sheet names or choosing specific columns to export, you enhance the presentation and utility of your Excel documents. With capabilities like ExcelWriter
, managing multiple sheets in a single workbook becomes efficient and organized. Harness these techniques to streamline your data export workflows, ensuring your data is conveyed clearly and effectively in the widely-used Excel format.
No comments yet.