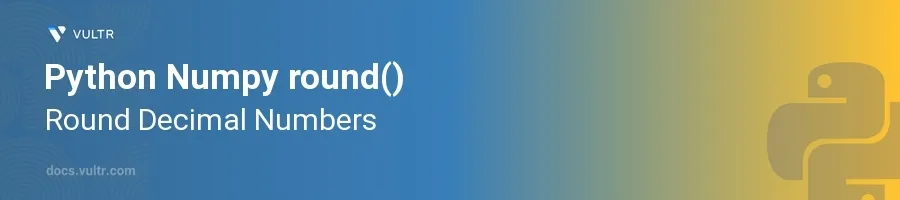
Introduction
The round()
function in NumPy is an essential tool for data scientists and analysts who frequently work with numerical data, especially when precision matters in their calculations and presentations. This function simplifies the process of rounding decimal numbers to a specified number of decimal places, ensuring consistency and precision in data outcomes.
In this article, you will learn how to efficiently utilize the round()
function within the NumPy library to handle decimal rounding operations. Discover how to execute basic rounding tasks and explore rounding in more complex scenarios, like multi-dimensional arrays and where specific rounding rules are required.
Basic Rounding of Decimal Numbers
Simple Rounding to Closest Integer
Import the NumPy library.
Create an array of floating-point numbers.
Use the
round()
function to round these numbers to the nearest integers.pythonimport numpy as np decimal_array = np.array([1.7, 2.3, 3.8, 4.1]) rounded_array = np.round(decimal_array) print(rounded_array)
This code results in a NumPy array where decimals are rounded to the nearest whole numbers:
[2., 2., 4., 4.]
.
Specifying Decimal Places
Define the number of decimal places to which you want to round.
Pass this value as the second argument to the
round()
function.pythondecimal_array = np.array([1.68, 2.34, 3.85, 4.19]) rounded_array = np.round(decimal_array, decimals=1) print(rounded_array)
In this example, each number in the array is rounded to one decimal place, resulting in:
[1.7, 2.3, 3.9, 4.2]
.
Advanced Rounding Techniques
Rounding Multi-Dimensional Arrays
Work with an array containing multiple dimensions.
Apply the
round()
function across the entire array.pythonmatrix = np.array([[2.65, 3.45], [4.75, 6.55]]) rounded_matrix = np.round(matrix, decimals=1) print(rounded_matrix)
Here, each element within the multi-dimensional array is rounded to one decimal place:
[[2.7, 3.4], [4.8, 6.6]]
.
Handling Complex Numbers
Understand that
round()
can also be applied to arrays of complex numbers by rounding both the real and imaginary parts separately.Define an array with complex numbers and apply rounding.
pythoncomplex_array = np.array([1.7+2.6j, 2.3+3.1j]) rounded_complex = np.round(complex_array, decimals=1) print(rounded_complex)
Rounding affects both the real and imaginary components, giving:
[1.7+2.6j, 2.3+3.1j]
.
Conclusion
The NumPy round()
function provides a robust way to handle numerical data with precision across various scenarios, including handling floating-point numbers, complex numbers, and multi-dimensional arrays. By mastering the rounding techniques demonstrated, maintain high accuracy and consistency in data processing tasks. Whether you're rounding for presentation clarity or computational needs, NumPy's rounding capabilities make it an indispensable tool in your Python data science toolkit.
No comments yet.