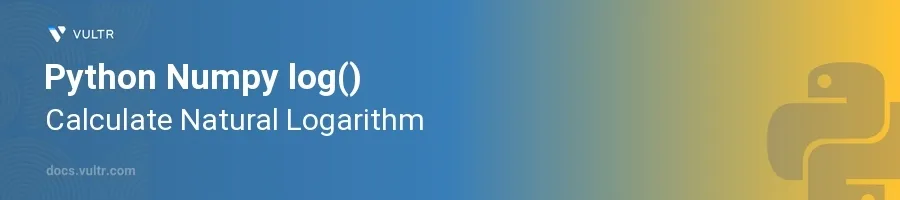
Introduction
The log()
function from the Numpy library in Python is a versatile tool for computing the natural logarithm of an array of numbers, where the natural logarithm is the logarithm to the base e
. This function is essential in scientific computing for growth calculations, time constants in physics, and in various fields of engineering and data science.
In this article, you will learn how to use the Numpy log()
function to compute natural logarithms for different types of numerical data. This includes handling single numbers, lists, and arrays, as well as managing special cases like zero or negative values.
Calculating Natural Logarithm of a Single Number
Compute Logarithm for Positive Numbers
Import the Numpy library.
Use
log()
to calculate the natural logarithm of a positive number.pythonimport numpy as np number = 5 log_result = np.log(number) print(log_result)
This code calculates the natural logarithm of the number
5
. The output will be approximately1.609
.
Handle Zero and Negative Numbers
Be aware that the natural logarithm is not defined for zero or negative numbers, and attempting to compute it will result in a runtime error.
Use error handling to manage attempts to calculate logarithms of non-positive numbers.
pythonimport numpy as np number = -1 try: log_result = np.log(number) print(log_result) except ValueError as e: print("Error: ", e)
This snippet catches the
ValueError
and prints an error message, which helps in debugging or user notification.
Working with Lists and Arrays
Calculate Logarithms for Each Element in a List
Create a list of positive numbers.
Convert the list to a Numpy array.
Apply
np.log()
to the entire array.pythonimport numpy as np num_list = [1, 2, 3, 4, 5] num_array = np.array(num_list) log_array = np.log(num_array) print(log_array)
Here,
np.log()
computes the natural logarithm for each element in thenum_array
, returning an array of logarithmic values.
Managing Special Values in Arrays
Recognize that Numpy can handle special values like NaN or infinity.
Process an array containing a mix of positive numbers and zero to observe output.
pythonimport numpy as np special_values = [1, 0, -1, np.inf] log_specials = np.log(special_values) print(log_specials)
This example demonstrates how Numpy returns
-inf
forlog(0)
and warns or throws an error for negative numbers.
Conclusion
The log()
function in Numpy is a powerful feature for computing natural logarithms, vital in various scientific and engineering tasks. By understanding how to properly apply this function to different data types and handle special cases, you can efficiently implement logarithmic calculations in your Python projects. Always ensure to manage non-positive inputs gracefully to maintain robustness in your numerical applications.
No comments yet.