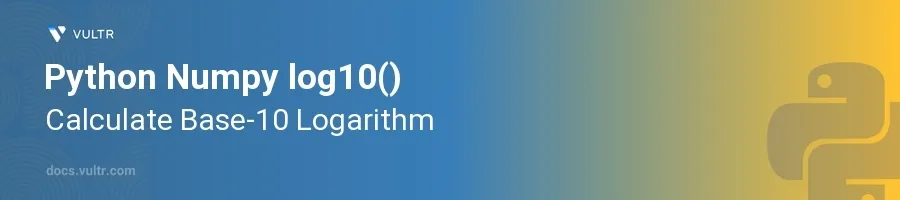
Introduction
The numpy.log10()
function in Python is crucial for scientific computing, particularly when dealing with logarithmic scales that require a base-10 logarithm. This function is part of the Numpy library, widely used in data analysis, engineering, and physics, to transform data to a logarithmic scale which can make patterns more visible and data more manageable.
In this article, you will learn how to utilize the numpy.log10()
function in different contexts. You will see how this function processes various numeric data types and structures, from single numbers to arrays, helping to facilitate data analysis and mathematical computations.
Using numpy.log10() with Single Numbers
Compute Base-10 Logarithm of a Single Number
Import the
numpy
library.Define a single numeric value.
Use
numpy.log10()
to compute the base-10 logarithm.pythonimport numpy as np number = 100 log_result = np.log10(number) print(log_result)
This example calculates the base-10 logarithm of 100, which equals 2. This is because (10^2 = 100).
Using numpy.log10() with Arrays
Calculate Base-10 Logarithms for Each Element in an Array
Ensure the
numpy
library is imported.Create a numpy array with multiple numbers.
Apply
numpy.log10()
to the entire array.pythonimport numpy as np numbers_array = np.array([1, 10, 100, 1000]) log_results = np.log10(numbers_array) print(log_results)
The function processes each element in the array
numbers_array
and returns an array of their base-10 logarithms. The results[0., 1., 2., 3.]
correspond to the logarithms (10^0, 10^1, 10^2, 10^3).
Handling Special Values and Errors
Managing Zero and Negative Inputs
Recognize that logarithms for zero or negative numbers are not defined in the real number system.
Pass a zero or negative number to
numpy.log10()
.Understand how Numpy handles these cases by returning NaN or infinities.
pythonimport numpy as np special_values = np.array([0, -1, -100]) log_special = np.log10(special_values) print(log_special)
The output demonstrates
numpy
s behavior with non-positive numbers, returning[-inf, NaN, NaN]
respectively, indicating errors and undefined values.
Conclusion
The numpy.log10()
function is an effective tool for performing base-10 logarithmic transformations on both single values and arrays in Python. This function is particularly useful in scientific and engineering disciplines where logarithmic data scaling is common. By mastering numpy.log10()
, you enhance your ability to process and analyze data, making it easier to uncover underlying patterns and relationships. Utilize the discussed approaches in your data handling and mathematical tasks to maintain precise and efficient computations.
No comments yet.