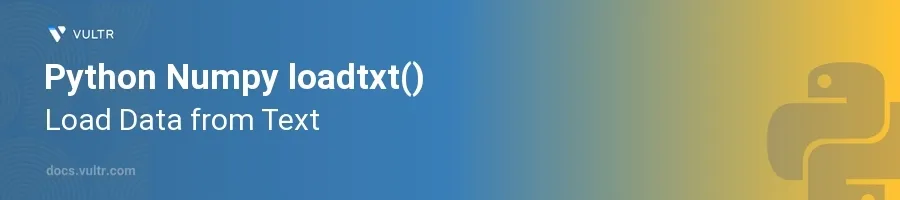
Introduction
The numpy.loadtxt()
function in Python provides a powerful yet simple way to read data from text files into a Numpy array. This utility is particularly valuable for scientists, engineers, and developers who frequently work with numerical data stored in text formats like CSV or TSV. It provides options to handle different data types and complex file structures efficiently.
In this article, you will learn how to leverage the loadtxt()
function to import data into Python efficiently. Understand how to handle various data formats, skip rows using the numpy loadtxt skiprows
function, use custom delimiters, and conduct basic processing during the load phase. These skills will equip you to manage and transform raw data into a usable format for analysis.
Basic Usage of NumPy loadtxt()
Load Data from a Simple Text File
Prepare a simple CSV text file with numerical data.
Use the
loadtxt()
function to read the file.pythonimport numpy as np data = np.loadtxt('path_to_file.csv', delimiter=',') print(data)
This code reads the CSV file specified by
'path_to_file.csv'
using a comma as the delimiter. The data is loaded into the arraydata
. This snippet demonstrates the basic functionality of the Numpy read text file operations.
Specify Data Types
Define the data type for the columns in the text file.
Use the
dtype
argument to specify the datatype while loading.pythondata = np.loadtxt('path_to_file.csv', delimiter=',', dtype=float) print(data)
Here, you ensure that the numbers are treated as floating point numbers, which can be crucial for maintaining precision in numerical computations. The
np.loadtxt dtype
argument allows explicit type definition to prevent unwanted type conversions.
Advanced Features of Numpy loadtxt()
Function
Skipping Header Rows
Identify the number of header rows in your file.
Use the
skiprows
argument to ignore the headers.pythondata = np.loadtxt('path_to_file.csv', delimiter=',', skiprows=1) print(data)
By specifying
skiprows=1
, the function skips the first row of the file. This is useful when dealing with files that include column headings or metadata in the initial rows. Thenumpy.loadtxt skiprows
feature here ensures that the code can efficiently handle structured files.
Handling Missing Data
Prepare for missing data by deciding on a filling strategy.
Utilize the
filling_values
parameter to handle any missing values.pythondata = np.loadtxt('path_with_missing_data.csv', delimiter=',', dtype=float, filling_values=0) print(data)
In this example, any missing values in 'path_with_missing_data.csv' are replaced with
0
, ensuring the array does not contain undefined values which could disrupt downstream processes.
Conclusion
The numpy.loadtxt()
function significantly simplifies the task of reading data from text files, especially for numerical data analysis. With its ability to handle various file formats, skip unnecessary rows, and replace missing values, loadtxt numpy
proves to be a convenient and robust solution for preparing raw data for further analysis. By mastering how to utilize numpy.loadtext
effectively, you streamline your data preparation workflows and ensure your data analysis projects are off to a good start.
No comments yet.