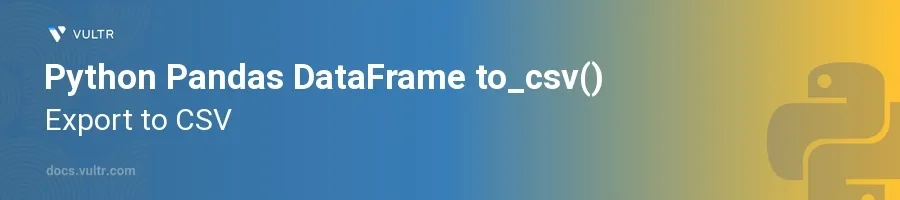
Introduction
The to_csv()
method in Python's Pandas library is essential for data analysts and programmers who need to export Pandas DataFrame to CSV files. This functionality allows for easy sharing and storage of large datasets in a universally compatible format. Whether you are preprocessing data for machine learning models, generating reports, or archiving historical records, understanding how to efficiently save Pandas DataFrame to CSV can enhance your data management capabilities.
In this article, you will learn how to use the to_csv()
method effectively to save a DataFrame as CSV in Python. Explore the options available for exporting DataFrame to CSV, managing special characters, handling large files, and ensuring data integrity during the export process.
Basics of DataFrame to CSV Conversion
Basic CSV Export
Import the Pandas library and create a DataFrame.
Use the
to_csv()
method to write Pandas DataFrame to CSV.pythonimport pandas as pd data = {'Name': ['John', 'Anna', 'Xiang'], 'Age': [28, 22, 29]} df = pd.DataFrame(data) df.to_csv('output.csv')
In this example, a simple DataFrame containing names and ages is created and exported to a CSV file named
output.csv
. The CSV file will automatically include headers and index numbers for each row.
Export Without Index
Often, the DataFrame index is irrelevant in the output file and can be omitted to keep the CSV clean.
Use the
index
parameter to prevent exporting the DataFrame index.pythondf.to_csv('output_no_index.csv', index=False)
Setting
index=False
removes the DataFrame index from the CSV, resulting in a cleaner file that only contains the data and header row.
Advanced CSV Export Options
Specifying Columns to Export
You may want to export only a subset of columns, especially in wide DataFrames.
Use the
columns
parameter to explicitly define which columns to include in the CSV.pythondf.to_csv('output_selected_columns.csv', columns=['Name'], index=False)
This command exports DataFrame to CSV with only the 'Name' column, making it useful for selective data sharing or storing.
Handling Special Characters and Encoding
Special characters in data can cause issues in CSV files if not properly handled.
Utilize the
encoding
parameter to manage character encoding, ensuring the CSV is readable and consistent across different platforms or applications.pythondf.to_csv('output_utf8.csv', encoding='utf-8')
This specification ensures that any special characters are correctly encoded using UTF-8, which is the most common character encoding standard.
Compression of Large Files
Large datasets can result in very large CSV files that are difficult to handle and share.
Use the
compression
parameter to specify that the output CSV should be compressed.pythondf.to_csv('output_compressed.csv', compression='infer')
Setting
compression='infer'
automatically applies the appropriate compression format based on the provided file extension (e.g.,.gzip
,.zip
). This optimizes the export DataFrame to CSV process, ensuring the exported file is compressed in size.
Conclusion
The to_csv()
function from Pandas is a powerful tool for saving a DataFrame to CSV in Python. It ensures that data is stored in a widely accessible format, enables smooth data exchange across systems, and improves analytical workflows. By mastering various parameters available in to_csv()
, you can optimize your Pandas save to CSV process to meet specific requirements related to file size, data formatting, and encoding.
Understanding how to export a DataFrame to CSV in Python allows you to handle different data structures efficiently. Implement these techniques in different scenarios to optimize Python DataFrame to CSV operations and improve overall efficiency in handling large datasets.
No comments yet.