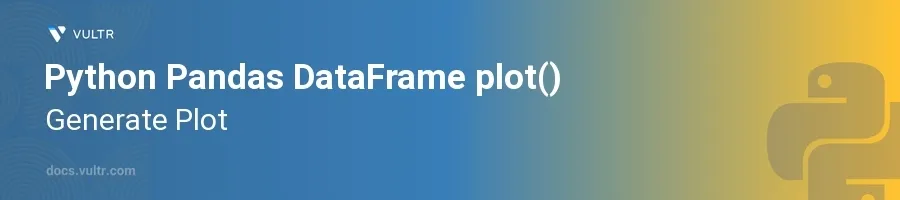
Introduction
The plot()
function in Python's Pandas library offers a versatile way to visualize data directly from DataFrame structures. This built-in function leverages the power of the popular plotting library Matplotlib, enabling users to create a variety of charts and graphs from their data seamlessly. Whether you need to display trends over time, relationships between variables, or distributions of data, the plot()
function provides an efficient gateway to visual analysis.
In this article, you will learn how to utilize the plot()
function to generate different types of visual outputs from a DataFrame. Explore how to customize plots with various parameters and see practical examples that illustrate the creation of line graphs, bar charts, histograms, and scatter plots.
Basic Usage of DataFrame.plot()
Generating a Simple Line Plot
Ensure you have the Pandas and Matplotlib libraries installed in your Python environment.
Import the necessary libraries.
Create a DataFrame.
Use the
plot()
function to generate a line plot.pythonimport pandas as pd import matplotlib.pyplot as plt # Sample data data = {'Year': [2010, 2011, 2012, 2013, 2014], 'Sales': [200, 300, 400, 500, 600]} df = pd.DataFrame(data) # Generating a line plot df.plot(x='Year', y='Sales', kind='line') plt.show()
This code creates a DataFrame
df
from a dictionary and then usesplot()
to create a line graph plotting 'Year' against 'Sales'. Thekind='line'
parameter specifies that a line plot should be generated.plt.show()
displays the plot.
Creating a Bar Chart
Use
plot()
with thekind
parameter set to'bar'
.Customize the plot with titles and labels.
python# Generating a bar chart df.plot(x='Year', y='Sales', kind='bar', title='Annual Sales', color='blue') plt.xlabel('Year') plt.ylabel('Sales') plt.show()
In this example,
kind='bar'
changes the plot type to a bar chart. Additional Matplotlib functions likeplt.xlabel()
andplt.ylabel()
are used to label the x-axis and y-axis, respectively.
Advanced Plotting Techniques
Plotting Multiple Columns
Add more data columns to the DataFrame.
Plot multiple columns in a single graph.
python# Adding more data data['Expenses'] = [150, 200, 250, 300, 350] df = pd.DataFrame(data) # Plotting multiple columns df.plot(x='Year', y=['Sales', 'Expenses'], kind='line') plt.title('Sales vs Expenses over Years') plt.show()
This snippet updates the DataFrame to include an 'Expenses' column and plots both 'Sales' and 'Expenses' over the years. Different lines on the same plot allow for easy comparison.
Creating Histograms
Consider using histograms to analyze data distributions.
Generate a histogram with the
plot()
function.python# Random data for histogram data = {'Values': [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]} df = pd.DataFrame(data) # Histogram df.plot(kind='hist', bins=4, alpha=0.7, color='green', title='Value Distribution') plt.xlabel('Values') plt.show()
The histogram created here highlights the distribution of values in the DataFrame. The
bins
parameter controls the number of bins used in the histogram, andalpha
determines the transparency of the bars.
Customizing Plots
Enhance plot aesthetics and readability by adding legends, changing colors, adjusting ticks, and more.
- Adding a Legend: Use
plt.legend()
to help identify plotted data series. - Changing Line Styles: Customize lines with colors, widths, and styles (e.g., dashed).
- Annotations: Employ
plt.text()
orplt.annotate()
to add text or annotations to specific points.
Conclusion
The plot()
function in the Pandas library simplifies the task of generating insightful graphical representations from DataFrame data. By mastering its use, you effectively translate raw data into visual formats that facilitate easier comprehension and analysis. From basic line charts to more complex histograms and scatter plots, grasp these techniques to enhance the analytical capabilities of your Python scripts. By following the examples provided, empower your data analysis processes with robust, visually engaging plots.
No comments yet.