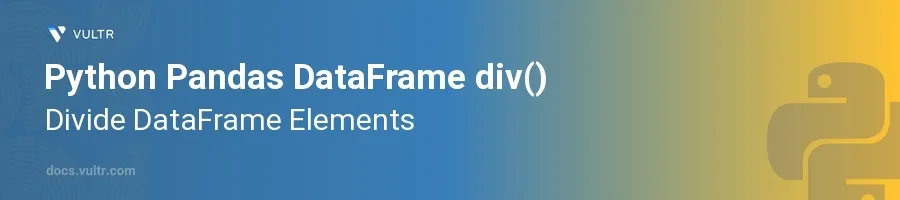
Introduction
In data analysis, division operations are frequently needed to normalize data, calculate ratios, or adjust features in datasets. The Pandas library in Python offers a robust toolset for managing such data operations efficiently using DataFrames. One such tool is the div()
method, which allows for element-wise division of DataFrame elements by another DataFrame, Series, or a scalar value.
In this article, you will learn how to use the div()
method in Pandas to perform various division tasks on DataFrame elements. Discover how to handle different scenarios such as dividing by another DataFrame, a Series, and a scalar value, along with understanding how to manage division by zero and other special cases.
Dividing by a Scalar
When performing operations across a dataset, you might need to scale down or adjust the data by a uniform scale, known as scalar. Here’s how to use div()
to divide each element of a DataFrame by a scalar.
Create your DataFrame.
Use the
div()
method with a scalar value.pythonimport pandas as pd # Sample DataFrame df = pd.DataFrame({ 'A': [10, 20, 30], 'B': [100, 200, 300] }) # Divide each element by 2 result = df.div(2) print(result)
This code divides each element of the DataFrame
df
by2
. It effectively performs a uniform division across all columns and rows.
Dividing DataFrame by Another DataFrame
Sometimes, division operations need to be performed element-by-element between two DataFrames of the same size. Here’s how to apply div()
in such cases.
Prepare two DataFrames of the same dimensions.
Use
div()
to divide the first DataFrame by the second.python# First DataFrame df1 = pd.DataFrame({ 'A': [20, 40, 60], 'B': [200, 400, 600] }) # Second DataFrame df2 = pd.DataFrame({ 'A': [2, 4, 6], 'B': [10, 20, 30] }) # Element-wise division of df1 by df2 result = df1.div(df2) print(result)
This code demonstrates element-wise division where each cell in
df1
is divided by the corresponding cell indf2
.
Dividing DataFrame by a Series
To apply row or column-wise operations, Pandas allows division of a DataFrame by a Series. Depending on the axis parameter (axis=0
for column-wise, axis=1
for row-wise), operations can be tailored.
Consider a DataFrame and a Series.
Apply the
div()
method, specifying the axis.python# DataFrame df = pd.DataFrame({ 'A': [20, 40, 60], 'B': [200, 400, 600] }) # Series series = pd.Series([2, 10]) # Divide DataFrame by Series along the columns result = df.div(series, axis=0) print(result)
This operation divides each column in the DataFrame
df
by the Seriesseries
, demonstrating a column-wise operation.
Handling Division by Zero
In any division task, division by zero might occur. It’s essential to handle these cases to prevent errors. Here’s how Pandas manages these situations.
Attempt to divide by zero and observe the outcome.
Utilize parameters to adjust the behavior.
python# Series with a zero value series = pd.Series([2, 0]) # Attempt division result = df.div(series, axis=0) print(result)
Pandas automatically handles division by zero by placing NaN (Not a Number) in locations where division by zero occurs.
Conclusion
By mastering the div()
method in Pandas, handling division operations across DataFrame elements becomes seamless and efficient. From simplifying data normalization tasks to performing complex element-wise calculations, div()
offers the robustness needed for precise data manipulation. Utilize this method in your data preparation or feature engineering stages to streamline the preparation of data for analytics or machine learning models. Equip yourself with these techniques to enhance the flexibility and efficiency of your data manipulation toolkit.
No comments yet.