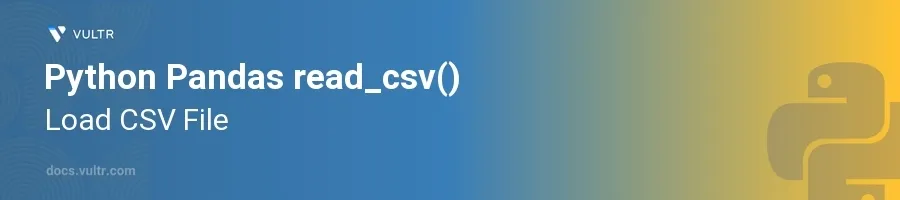
Introduction
The read_csv()
function from the Pandas library in Python is a crucial tool for data analysts and scientists. This function allows users to easily import CSV (Comma Separated Values) files into DataFrame objects, facilitating data manipulation and analysis with Pandas. The versatility and efficiency of read_csv()
make it an essential component for any data-driven Python project.
In this article, you will learn how to employ the read_csv()
function to load CSV files into DataFrames effectively. You will explore various parameters that can be tailored to address different data characteristics and ensure seamless data loading and preprocessing.
Basic Usage of read_csv()
Load a Simple CSV File
Start by importing the Pandas library.
Use
read_csv()
to load a CSV file into a DataFrame.pythonimport pandas as pd df = pd.read_csv('path/to/your/file.csv') print(df.head())
Immediately upon execution, this code reads the CSV file located at 'path/to/your/file.csv' and loads the data into Pandas DataFrame
df
.df.head()
displays the first five rows of the DataFrame.
Specify Index Column
Identify a column in the CSV file that should be used as the index of the DataFrame.
Use the
index_col
argument to specify the index column.pythondf = pd.read_csv('path/to/your/file.csv', index_col='ID') print(df.head())
Specifying the
index_col
as 'ID' conditionsread_csv()
to use the 'ID' column from the CSV as the DataFrame’s index column.
Handling Missing Data
Deal with Missing Values
Understand how Pandas handles missing values by default (typically represented as
NaN
).Utilize parameters like
na_values
to customize how missing values are recognized in the CSV.pythondf = pd.read_csv('file.csv', na_values=['NA', 'n/a', 'not available'])
This line directs
read_csv()
to interpret 'NA', 'n/a', and 'not available' as missing values, converting them intoNaN
.
Advanced Data Parsing Options
Parse Dates
Recognize the need to parse columns that contain dates during the loading process.
Use the
parse_dates
parameter to specify columns that should be parsed as dates.pythondf = pd.read_csv('file.csv', parse_dates=['Date_Column'])
Setting
parse_dates
to['Date_Column']
ensures that the 'Date_Column' in the CSV is parsed as a date, significantly simplifying future time-series operations.
Use Custom Delimiters
Identify when CSV files utilize delimiters other than commas (e.g., tabs, semicolons).
Leverage the
sep
parameter to define the correct delimiter.pythondf = pd.read_csv('file_with_tabs.csv', sep='\t')
In this snippet,
sep='\t'
teachesread_csv()
to treat tabs (\t
) as delimiters, catering to CSV files that use tabs to separate the data fields.
Handling Large Data Files
Efficient Reading of Large Files
Address potential memory issues when loading large CSV files.
Use the
chunksize
parameter to read the file in smaller chunks, ornrows
to limit the number of rows read.pythonchunk_iter = pd.read_csv('large_file.csv', chunksize=1000) for chunk in chunk_iter: print(chunk.head())
The
chunksize
parameter ensures thatread_csv()
processes the file in segments containing 1000 rows each, conserving memory and enhancing performance.
Conclusion
Mastering the read_csv()
function in Pandas equips you with the ability to handle a wide variety of data loading scenarios efficiently. From basic file reading to advanced data preparation tasks, this function serves as a cornerstone in Python's data analysis endeavors. By tuning parameters such as index_col
, na_values
, parse_dates
, and chunksize
, tailor the data loading process to fit the specific needs of any project, leading to more streamlined and effective data analysis workflows.
No comments yet.