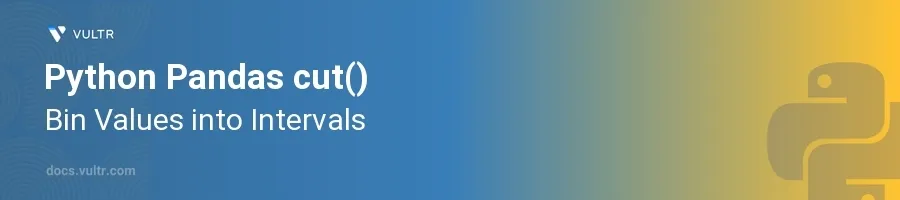
Introduction
The cut()
function in Python's Pandas library serves as a utility to segment and sort data values into bins or intervals. This functionality comes in handy especially when dealing with data analysis, where creating categorical variables from a continuous feature is necessary to simplify the analysis or to divide a dataset into perceptive groups.
In this article, you will learn how to harness the cut()
function to efficiently categorize continuous data into intervals. Explore the fundamentals of creating bins, assigning labels, and dealing with edge cases. Additionally, the discussion will cover examples illustrating practical applications of cut()
in data preprocessing tasks.
Understanding the cut() Function
The function pandas.cut()
is used to bin continuous data into discrete intervals, which can help in transforming continuous variables into categorical variables. This type of data transformation is particularly useful in various sectors, such as financial analysis, market segmentation, and scientific research. Here’s how you can effectively use the cut()
function.
Basic Binning: Divide Data into Equal Ranges
Import the Pandas library.
Create or import a series of continuous data.
Define the number of bins or explicitly specify the bin edges.
Apply the
cut()
function to the data.pythonimport pandas as pd data = pd.Series([1, 2, 13, 19, 24, 30]) bins = pd.cut(data, bins=3) print(bins)
This snippet creates 3 equal width bins from the data in the series. The resulting bins divide the range of data into intervals that you can further analyze or use in your data models.
Adding Labels to Bins
Define a list of labels that corresponds to the number of bins.
Apply the
cut()
function with the labels option.pythonlabels = ['Low', 'Medium', 'High'] labeled_bins = pd.cut(data, bins=3, labels=labels) print(labeled_bins)
With labels added, each item in the series is not just categorized into a bin but is also assigned a descriptive label, which can improve the interpretability of the results.
Handling Edge Cases
Consider the minimum and maximum values of your data.
Decide whether to include these values within the first or last bins.
Use the
include_lowest
parameter to customize edge inclusion.pythonbins_edge = pd.cut(data, bins=3, include_lowest=True) print(bins_edge)
This code ensures that the lowest boundary is inclusive, meaning that values exactly on the lower boundary of the first bin are included within that bin. Alternatively, you may also use
right=False
to include the upper boundary of the last bin.
Special Considerations for Non-Uniform Bin Sizes
Sometimes, equal intervals might not suit your analysis, especially if the data is skewed or if you need bins of different sizes.
Define an array of bin edges.
Use this array in the
cut()
function.pythoncustom_bins = [0, 5, 15, 20, 30] non_uniform_bins = pd.cut(data, bins=custom_bins) print(non_uniform_bins)
In this example, the bins are manually defined with specific ranges, allowing for flexibility in how the data is categorized.
Evaluating Utilization of cut() in Real-world Scenarios
Implementing the cut()
function extends beyond basic examples. In real-world scenarios, such as consumer behavior analysis or demographic studies, the cut()
function contributes significantly to data segmentation. Manage larger datasets effectively by categorizing data, which can help in creating focused, strategic insights.
Conclusion
The cut()
function in Pandas allows you to bin numerical data into insightful categories or intervals, enhancing your data analysis processes. Start utilizing cut()
to categorize your continuous data effectively, integrate labels smoothly, and handle edges meticulously. Employ these techniques in your data analysis practices to optimize your workflow, improve data visualization, and provide clearer interpretations of data trends and distributions. Familiarize yourself with options like bin sizes and labels to maximize the utility of your categorized data.
No comments yet.