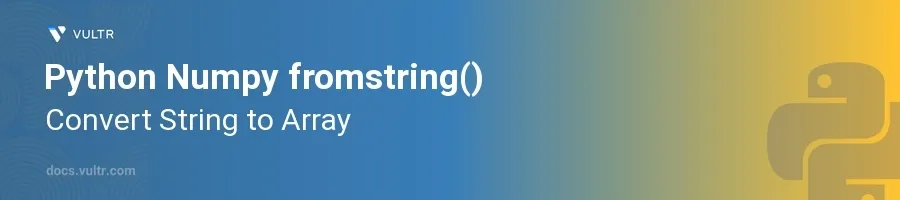
Introduction
The fromstring()
function in Python's NumPy library is a powerful tool for converting string data into numerical arrays quickly and efficiently. This function primarily serves to parse a string of numbers separated by a delimiter and convert it into an array of a specified data type, which is crucial for handling large datasets or transforming data read from text files.
In this article, you will learn how to leverage the fromstring()
function to transform string data into NumPy arrays. Explore various examples that demonstrate how this function handles different types of data inputs and delimiters, and learn how to effectively implement it in your data processing workflows.
Basic Usage of fromstring()
Convert a Simple String to Array
Start with a basic string representing numerical values separated by commas.
Convert the string to a NumPy array using
fromstring()
specifying the data type and delimiter.pythonimport numpy as np data = "1,2,3,4,5" array = np.fromstring(data, dtype=int, sep=',') print(array)
This code converts the string
data
into an array of integers. Thesep=','
argument tellsfromstring()
that the numbers in the string are separated by commas.
Managing Different Delimiters
Define a string with numbers separated by semicolons.
Use
fromstring()
to process this data by specifying the semicolon as the delimiter.pythondata = "1;2;3;4;5" array = np.fromstring(data, dtype=int, sep=';') print(array)
Here,
fromstring()
correctly interprets the semicolon-separated numbers, effectively creating an integer array.
Advanced Use Cases
Handling Decimal Values
Consider a string of decimal values, potentially separated by spaces.
Process these values into a floating-point array.
pythondata = "1.1 2.2 3.3 4.4 5.5" array = np.fromstring(data, dtype=float, sep=' ') print(array)
In this example, each space-separated value in
data
is converted into a float, which can be particularly useful when dealing with decimal numbers in data analysis.
Reading Hexadecimal Values
Illustrate a scenario where hexadecimal numbers stored as a string need to be converted.
Use
fromstring()
to read these hexadecimal numbers into an integer array.pythondata = "1a 2b 3c 4d 5e" array = np.fromstring(data, dtype='int', sep=' ') print(array)
The function interprets the hexadecimal values (given the correct context in actual use might require additional steps) and converts them to integers.
Conclusion
The fromstring()
function in NumPy is an essential tool for transforming strings into arrays efficiently, supporting various data types and delimiters. By integrating this function into your data processing routines, streamline workflows involving data conversion and manipulation. By mastering the techniques discussed, make sure your array manipulations are both effective and efficient, aiding significantly in data-driven programming tasks.
No comments yet.