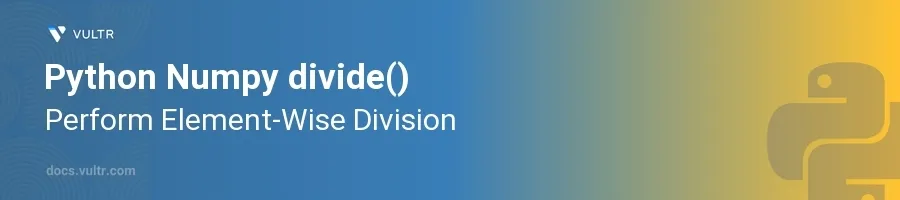
Introduction
The numpy.divide()
function is an essential tool in numerical computing, especially when dealing with arrays and matrices in Python. This function facilitates element-wise division of array elements, allowing for the efficient computation of division operations across large datasets or complex numerical problems.
In this article, you will learn how to effectively utilize the numpy.divide()
function for various applications. Explore practical scenarios where this function can be applied to simplify the division operations in multi-dimensional arrays, handling different data types, and ensuring code adaptability across multiple projects.
Basics of numpy.divide()
Syntax and Parameters
- Gain an understanding of the basic syntax of
numpy.divide()
:pythonnumpy.divide(x1, x2, out=None, where=True, casting='same_kind', order='K', dtype=None, subok=True)
x1
,x2
: Input arrays to be divided.x1
is the dividend andx2
is the divisor.out
: Optional. An alternate output array in which to place the result.where
: Optional. A condition on which to broadcast the operation.- Other parameters like
casting
,order
,dtype
, andsubok
help define how the operation should be performed and the return type.
Simple Example: Dividing Two Arrays
Initialize two numpy arrays.
Apply the
divide()
function.pythonimport numpy as np arr1 = np.array([10, 20, 30, 40]) arr2 = np.array([2, 2, 2, 2]) result = np.divide(arr1, arr2) print(result)
This code snippet will output the result of dividing each element of
arr1
by the corresponding element inarr2
, yielding[5.0, 10.0, 15.0, 20.0]
.
Handling Different Data Types
Float and Integer Division
Understand the impact of data types in division operations.
Use
numpy.divide()
with a mix of float and integer data types.pythonarr1 = np.array([10.0, 20.0, 30.0, 40.0]) # float array arr2 = np.array([2, 3, 4, 5]) # integer array result = np.divide(arr1, arr2) print(result)
Here, floating-point division happens due to mixed data types, and the output is
[5.0, 6.66666667, 7.5, 8.0]
. Notice the float result owing to the floating-point type ofarr1
.
Advanced Usage
Broadcasting and Conditional Divisions
Broadcast operations when dividing arrays of different sizes.
Include conditions to selectively perform division.
pythonarr1 = np.array([[20, 30, 40], [50, 60, 70]]) arr2 = np.array([2, 3, 5]) result = np.divide(arr1, arr2, where=arr1>30) print(result)
This example demonstrates broadcasting and conditional division where the division will only apply to elements of
arr1
that are greater than 30. Non-qualified elements will remain unchanged.
Conclusion
Using numpy.divide()
in Python significantly simplifies element-wise division across arrays and matrices, enhancing the capability to handle diverse computational problems efficiently and effectively. Whether dealing with simple arrays or complex broadcasting requirements, numpy.divide()
offers flexible, powerful solutions. By familiarizing yourself with its parameters and capabilities, you ensure your numerical computations are both robust and efficient.
No comments yet.