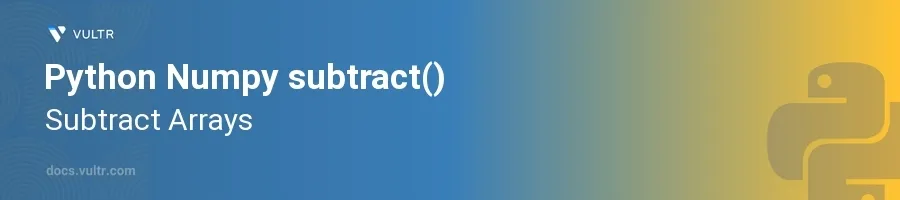
Introduction
Numpy is a fundamental package for scientific computing in Python, providing support for large, multi-dimensional arrays and matrices along with a large collection of high-level mathematical functions to operate on these arrays. One such function is subtract()
, which simplifies the process of element-wise subtraction between two arrays. This function is essential for data manipulation and computational tasks where precise control over arithmetic operations is required.
In this article, you will learn how to effectively use the subtract()
function in various contexts. Discover the versatility of this method in handling both basic and complex array operations, enhancing code readability and efficiency in your data processing workflows.
Understanding Numpy subtract()
Basic subtraction of Two Arrays
Make sure you have two arrays of the same shape.
Use
numpy.subtract()
to perform element-wise subtraction.pythonimport numpy as np arr1 = np.array([10, 20, 30]) arr2 = np.array([1, 2, 3]) result = np.subtract(arr1, arr2) print(result)
This code subtracts each element in
arr2
from the corresponding element inarr1
. Theresult
array would output[9, 18, 27]
.
Subtracting Scalars from an Array
Initialize an array and a scalar value.
Apply
numpy.subtract()
to reduce the scalar from each array element.pythonarr = np.array([5, 10, 15]) scalar = 3 result = np.subtract(arr, scalar) print(result)
Here,
3
is subtracted from each element in the arrayarr
, resulting in the array[2, 7, 12]
.
Using subtract() with Broadcasting
Understand Numpy broadcasting rules when working with arrays of different shapes.
Create two arrays where one array has a dimension size of
1
.Use
numpy.subtract()
to perform subtraction.pythonarr1 = np.array([[10, 20, 30]]) arr2 = np.array([[1], [2], [3]]) result = np.subtract(arr1, arr2) print(result)
In this example,
arr1
is a 1x3 array andarr2
a 3x1 array. Numpy automatically broadcasts these arrays to a common shape for element-wise subtraction, resulting in a 3x3 array:[[ 9 19 29] [ 8 18 28] [ 7 17 27]]
In-Place Subtraction for Memory Efficiency
Start with initializing your main array and the subtracting array or scalar.
Use
subtract()
without
parameter to perform the operation in-place.pythonarr1 = np.array([10, 20, 30]) arr2 = np.array([1, 2, 3]) np.subtract(arr1, arr2, out=arr1) print(arr1)
Setting
out=arr1
directsnumpy
to store the result inarr1
itself, thus saving memory by not creating a new array. Thearr1
will reveal[9, 18, 27]
after execution.
Advanced Techniques using subtract()
Handling Overflows and Data Types
Be aware of the data types of the arrays to prevent overflow.
Choose an appropriate data type to ensure accurate computations.
pythonarr1 = np.array([30000], dtype=np.int16) arr2 = np.array([10000], dtype=np.int16) result = np.subtract(arr1, arr2) print(result) print(result.dtype)
This subtraction could potentially cause overflow if not handled with the correct data type. Here,
result
is an array of typeint16
. Without proper type, the results may wrap around misleading values.
Utilizing Subtract in Image Processing
Manipulate values in image matrices to adjust brightness or perform other corrections.
Convert images into Numpy arrays and use
subtract()
for real-time adjustments.pythonimport imageio img = imageio.imread('path_to_image.jpg') darker_img = np.subtract(img, 50) # making the image darker imageio.imwrite('darker_image.jpg', darker_img)
This operation decreases the brightness of every pixel by 50 units, effectively making the image darker, which can be critical in image processing tasks like adjusting exposure.
Conclusion
The numpy.subtract()
function in Python plays a crucial role in array manipulations involving subtraction, from straightforward operations to complex broadcasted and in-place subtractions. By mastering this function, streamline numeric computations in scientific research, data analysis, and even in fields like image processing. With the examples discussed, gain proficiency in optimizing your array-based operations while ensuring efficiency and accuracy in your numerical computations.
No comments yet.