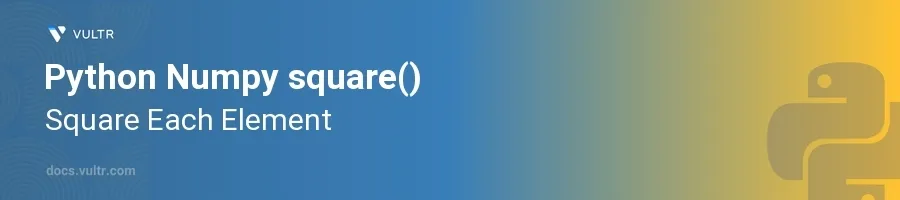
Introduction
The numpy.square()
function in Python is a straightforward and efficient method for squaring each element in an array. This capability is part of the Numpy library, which is central to scientific and numerical computing in Python. By squaring elements, you can perform a wide range of mathematical computations that are essential in fields like data analysis, engineering, and machine learning.
In this article, you will learn how to utilize the numpy.square()
function effectively. Explore how this function works on different types of numerical data, including integers, floats, and negatives, and see how it can be applied to both single-dimensional and multi-dimensional arrays.
The Basics of numpy.square()
Squaring Single-Dimensional Arrays
Install and import the Numpy library if you haven't already.
pythonimport numpy as np
Create a one-dimensional numpy array.
pythonarray_1d = np.array([1, 2, 3, 4, 5])
Use the
square()
function to square each element in the array.pythonsquared_array = np.square(array_1d) print(squared_array)
This code will output a new array with the squares of the original elements:
[1, 4, 9, 16, 25]
.
Applying the Function to Negative and Float Values
Understand that
numpy.square()
is capable of handling negative numbers and float values effectively.Create an array that includes both negative integers and floats.
pythonarray_mix = np.array([-1, -2.5, 3, 4.5])
Apply the
numpy.square()
function.pythonsquared_mix = np.square(array_mix) print(squared_mix)
This yields
[1.00, 6.25, 9.00, 20.25]
, demonstrating how the function handles different data types within an array.
Advanced Uses in Multi-Dimensional Arrays
Squaring Elements in 2D Arrays
Multi-dimensional arrays, or matrices, can also be processed using the
square()
function.Define a two-dimensional array.
pythonarray_2d = np.array([[1, 2], [3, 4]])
Use the
numpy.square()
function on the 2D array.pythonsquared_2d = np.square(array_2d) print(squared_2d)
The output,
[[ 1 4] [ 9 16]]
, shows the function's effective handling of squaring operations across multi-dimensional data structures.
Applying Square Function Across Different Axes
The
numpy.square()
function applies to the entire dataset by default, but manipulation along specific axes can be done through additional numpy functions likenp.sum()
if needed.Assume an operation involves squaring the elements and then summing them along rows.
pythonnp.sum(np.square(array_2d), axis=1)
This operation first squares each element in the matrix
array_2d
, and then sums these values across rows (axis 1).
Conclusion
The numpy.square()
function is a fundamental tool for element-wise operations in Python's Numpy library. It facilitates efficient computations where elements of an array are squared, supporting both simple and complex numerical tasks. This function seamlessly integrates into broader scientific and computational workflows, proving to be reliable and versatile in various applications. By mastering the practical uses demonstrated, harness the power of numpy.square()
to simplify mathematical operations and enhance the performance of your data processing tasks.
No comments yet.