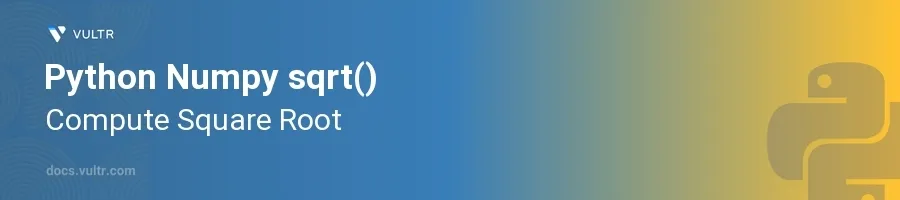
Introduction
The numpy.sqrt()
function in Python is a crucial tool for scientific and mathematical computations, allowing for the efficient calculation of square roots of elements in an array. This function is integral to the NumPy library, which is widely used in numerical data operations and scientific computing.
In this article, you will learn how to leverage the numpy.sqrt()
function in various scenarios, including handling arrays with both positive and negative numbers, as well as dealing with complex numbers. This guide will help you understand the function's versatility and how it can be applied in data analysis and scientific computations.
Basic Usage of numpy.sqrt()
Compute Square Root of Positive Numbers
Import the NumPy module.
Create an array of positive numbers.
Apply the
numpy.sqrt()
function.pythonimport numpy as np pos_array = np.array([4, 16, 25, 36]) sqrt_array = np.sqrt(pos_array) print(sqrt_array)
This snippet computes the square root of each number in
pos_array
. The output will be an array of the square roots:[2.0, 4.0, 5.0, 6.0]
.
Handling Non-Negative Numbers
Confirm understanding that
numpy.sqrt()
cannot process negative real numbers directly and returns NaN (Not a Number) by default.Create an array with non-negative integers to observe handling by NumPy.
pythonneg_array = np.array([1, -4, 9, -16]) sqrt_neg_array = np.sqrt(neg_array) print(sqrt_neg_array)
The output will show
NaN
for negative numbers:[1.0, NaN, 3.0, NaN]
.
Advanced Applications
Using numpy.sqrt() with Complex Numbers
Acknowledge that NumPy can compute the square roots of complex numbers.
Create an array of complex numbers.
Apply
numpy.sqrt()
to the array.pythoncomplex_array = np.array([1+1j, -1-1j, 4+4j]) sqrt_complex = np.sqrt(complex_array) print(sqrt_complex)
The computation handles complex numbers smoothly, returning square roots in complex form. This functionality is crucial for scientific fields where complex numbers often occur.
Applying numpy.sqrt() in Data Analysis
Utilize
numpy.sqrt()
to calculate derived data fields like the standard deviation.Create a dataset and calculate the mean.
Calculate the deviations from the mean, square them, and compute the square root of the mean square deviation (standard deviation).
pythondata = np.array([2, 4, 6, 8]) mean = np.mean(data) deviations = data - mean variance = np.mean(deviations ** 2) std_deviation = np.sqrt(variance) print(std_deviation)
Here
numpy.sqrt()
is used to compute the standard deviation from the variance, which is a fundamental task in statistical data analysis.
Conclusion
The numpy.sqrt()
function in Python is a dynamic tool that enhances the numerical and scientific computation capabilities of NumPy. Understanding its ability to process and return the square roots of real, non-negative, and complex numbers equips you with the resources to tackle advanced mathematical problems in your programming tasks. Utilize this function to manage calculations more robustly and efficiently in your data analysis and scientific computations. By mastering the techniques covered, you can ensure your applications are not only accurate but also performant.
No comments yet.