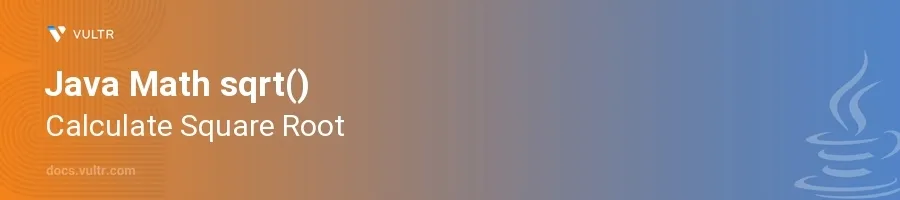
Introduction
The Math.sqrt()
method in Java is crucial for calculating the square root of a number. This method is frequently employed across various fields such as mathematics, engineering, science, and finance, where quick and accurate square root in Java calculations are essential.
In this article, you will learn how to use the Math.sqrt()
method efficiently in Java. Explore its application through practical examples that demonstrate its usage with different data types and scenarios, ensuring you can integrate this function seamlessly into your Java applications. Whether you're interested in sqrt in Java or need to understand math.sqrt
Java more deeply, this article has you covered.
Understanding Math.sqrt() in Java
Learn how to do square root in Java using the powerful Math.sqrt()
function in Java. This article will guide you exactly how to use Math.sqrt()
in Java to calculate square roots easily. With simple explanations and practical examples, you'll learn how to calculate square root in Java accurately and apply it confidently in your projects.
Basic Usage of Java Math.sqrt()
Declare a double variable.
Assign it a numeric value.
Use the
Math.sqrt()
method to calculate the square root.javadouble number = 9; double result = Math.sqrt(number); System.out.println("Square root of " + number + " is " + result);
This code calculates the square root of
9
, which results in3.0
.
Handling Negative Inputs
Recognize that
Math.sqrt()
returnsNaN
when passed a negative number.Check the input before applying
Math.sqrt()
.javadouble negativeNumber = -16; double result; if (negativeNumber < 0) { result = Double.NaN; System.out.println("Square root of negative number is not defined."); } else { result = Math.sqrt(negativeNumber); } System.out.println("Square root: " + result);
If the input is negative, this code correctly avoids using
Math.sqrt()
and informs the user that the square root of a negative number is not defined.
Calculating Square Roots in a Loop
Use
Math.sqrt()
in a loop to calculate and print square roots of an array of numbers.Iterate through the array, applying the square root method to each element.
javadouble[] numbers = {0, 1, 4, 9, 16, 25}; for (double num : numbers) { double sqrtValue = Math.sqrt(num); System.out.println("Square root of " + num + " is " + sqrtValue); }
This snippet will calculate and print the square roots of numbers in the array
numbers
.
Special Considerations
Precision and Data Types
Understand that
Math.sqrt()
is precise within the limits of double precision.Acknowledge that for very large numbers, the precision might not be as high.
javadouble largeNumber = 1e308; double result = Math.sqrt(largeNumber); System.out.println("Square root of very large number: " + result);
This example demonstrates how
Math.sqrt()
handles a very large number within the bounds of double precision.
Using Math.sqrt() with BigDecimal
Utilize
BigDecimal
for operations needing high precision over very large scales.Convert
BigDecimal
todouble
, applyMath.sqrt()
, then convert back if necessary.javaBigDecimal bigDecimalValue = new BigDecimal("12345.6789"); double sqrtDouble = Math.sqrt(bigDecimalValue.doubleValue()); BigDecimal sqrtResult = BigDecimal.valueOf(sqrtDouble); System.out.println("Square root of " + bigDecimalValue + " is " + sqrtResult);
Here the code transitions between
BigDecimal
anddouble
to applyMath.sqrt()
effectively while attempting to maintain precision.
Conclusion
The Math.sqrt()
function in Java efficiently computes the square root of a number and is essential in various professional fields requiring mathematical calculations. By understanding how to handle different data types and scenarios meticulously, ensure the correct implementation of this function to achieve high precision and reliability in square root calculations. With the examples and considerations discussed, you can now proficiently integrate the Math.sqrt()
method into your Java projects.
No comments yet.