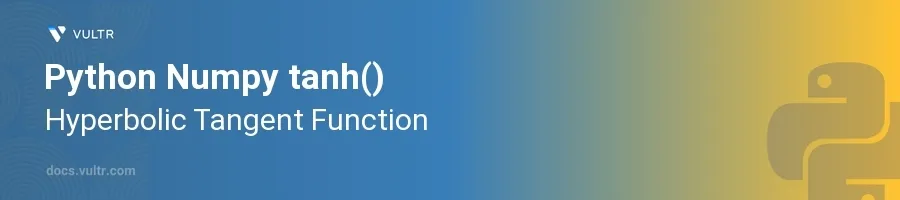
Introduction
The tanh()
function in Python, provided by the NumPy library, computes the hyperbolic tangent of an array of numbers. It serves as an essential tool in various scientific computing tasks, especially in the fields of machine learning and neural networks, where activation functions like the hyperbolic tangent are crucial. The function returns values between -1 and 1, providing smooth and efficient transitions in data transformation.
In this article, you will learn how to effectively employ the numpy.tanh()
function in your Python applications. Explore how to apply this function on arrays and matrices, handle special cases such as high and low input values, and integrate it into practical mathematical and machine learning tasks.
Utilizing numpy.tanh() in Arrays
Apply tanh() on a Single Dimensional Array
Import the NumPy library.
Create a one-dimensional array of numerical values.
Apply the
numpy.tanh()
function to the array.pythonimport numpy as np data = np.array([-3, -2, -1, 0, 1, 2, 3]) tanh_result = np.tanh(data) print(tanh_result)
This code calculates the hyperbolic tangent of each element in the array
data
. Thenumpy.tanh()
function processes element-wise, transforming each input to its respective hyperbolic tangent output.
Application in Two Dimensional Arrays
Define a two-dimensional array or matrix.
Execute
numpy.tanh()
on this matrix.pythonmatrix_data = np.array([[0, 0.5], [1, -1]]) tanh_matrix_result = np.tanh(matrix_data) print(tanh_matrix_result)
Applying
numpy.tanh()
on matrices processes each element individually, providing a transformed matrix where each original value is replaced with its hyperbolic tangent.
Practical Uses in Computational Tasks
Normalizing Data
Recognize that data normalization is crucial for algorithms requiring a normalized range.
Use the
tanh()
as a normalization function where data values need to be between -1 and 1.Normalize an array of values using
tanh()
.pythonraw_scores = np.array([10, 20, 30, -10, -20, -30]) normalized_scores = np.tanh(raw_scores) print(normalized_scores)
This example demonstrates how
numpy.tanh()
can effectively scale a wide range of values into a constrained range of [-1, 1], beneficial for models and algorithms in machine learning that are sensitive to the scale of input features.
Activation Function in Neural Networks
Understand that in neural networks, activation functions like
tanh()
help model complex patterns by adding non-linearity.Use
numpy.tanh()
in the context of simulating neurons in a neural network layer.Here,
numpy.tanh()
is used as an activation function for a layer of artificial neurons, taking the weighted sum of inputs and normalizing them into output signals that range from -1 to 1. This adds non-linear properties to the network, enabling it to learn and perform more complex tasks.Implementing neural networks often leverages libraries like TensorFlow or PyTorch, but understanding the role of
numpy.tanh()
within these frameworks is an essential foundational concept.
Handling Edge Cases
Dealing with High Input Values
Consider inputs with large magnitudes, where
tanh()
approaches -1 or 1 asymptotically.Observe how these edge cases are handled through the function.
When working with extremely high or low values,
numpy.tanh()
effectively caps these at near -1 or 1 respectively due to the properties of the hyperbolic tangent function. This behavior is particularly useful in avoiding issues related to numerical overflow and ensuring stability in calculations.
Conclusion
The numpy.tanh()
function is a versatile tool for data processing, especially beneficial in fields requiring smooth and bounded continuous functions, such as neural networks and other machine learning models. By understanding and implementing the uses of this function in various dimensions and applications, you enhance your capability to tackle complex mathematical and computational challenges effectively. Utilize the hyperbolic tangent function to optimize the performance and reliability of your scientific and machine learning applications, making the most of its fast, efficient, and reliable properties in numerical computing.
No comments yet.