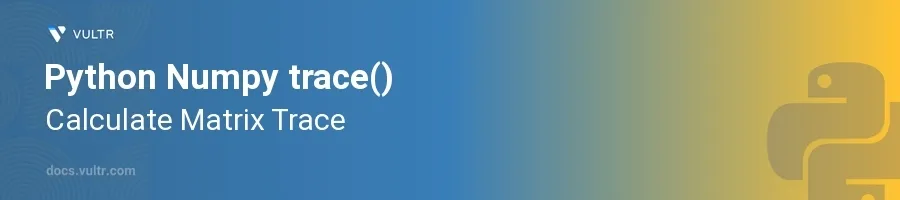
Introduction
The trace()
function in NumPy is an essential tool for mathematics and engineering applications, particularly when dealing with matrices. In linear algebra, the trace of a matrix is the sum of the diagonal elements and has several important properties and uses, including invariants under basis changes. This function simplifies the process of calculating the trace, offering a straightforward approach without manually summing these elements.
In this article, you will learn how to effectively utilize the trace()
function to calculate the trace of matrices using NumPy. The discussion will explore basic usage on square matrices, as well as variations such as using offsets and handling higher-dimensional arrays.
Calculating Trace of a Square Matrix
Basic Trace Calculation
Import the NumPy library.
Create a square matrix.
Use the
trace()
function to calculate the trace of the matrix.pythonimport numpy as np matrix = np.array([[1, 2], [3, 4]]) trace_value = np.trace(matrix) print(trace_value)
This code segment initializes a 2x2 matrix and computes its trace, which would be the sum of the diagonal elements
1
and4
, resulting in5
.
Handling Larger Matrices
Construct a larger matrix to demonstrate scalability.
Apply the
trace()
function.pythonlarge_matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) trace_large = np.trace(large_matrix) print(trace_large)
In this example, the trace calculation involves a 3x3 matrix. The trace is the sum of
1
,5
, and9
, which equals15
.
Utilizing Trace with Offset
Calculating Trace with Positive Offset
Signify importance of offset in the trace calculation.
Generate a matrix and calculate the trace with a positive offset.
pythonoffset_matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) trace_offset_positive = np.trace(offset_matrix, offset=1) print(trace_offset_positive)
By setting the
offset=1
, the trace function sums the elements just above the main diagonal, thus adding2
and6
, resulting in8
.
Calculating Trace with Negative Offset
Recognize that negative offset calculates the sum below the diagonal.
Apply the
trace()
function with a negative offset.pythontrace_offset_negative = np.trace(offset_matrix, offset=-1) print(trace_offset_negative)
When
offset=-1
, the function computes the sum of the elements4
and8
from below the main diagonal, giving a total of12
.
Working with Higher-dimensional Arrays
Trace Calculation in 3D Arrays
Understand that
trace()
can operate within higher-dimensional settings.Create a 3-dimensional array and compute traces on specified axes.
pythonthree_d_matrix = np.random.rand(2, 3, 3) # Two 3x3 matrices trace_3d = np.trace(three_d_matrix, axis1=1, axis2=2) print(trace_3d)
This snippet illustrates the computation of traces for each 3x3 matrix along the last two axes in a 3D matrix. The function returns a vector with the traces of each 2D matrix slice.
Conclusion
The trace()
function in Python's NumPy library is highly efficient for calculating the trace of matrices, a fundamental operation in various fields of mathematics and engineering. With the ability to handle not only simple matrices but also complex multidimensional arrays and offsets, it offers flexibility unmatched by manual computations. By mastering these techniques, you ensure accurate and efficient mathematical computations, fostering better analysis and results in your projects and research.
No comments yet.