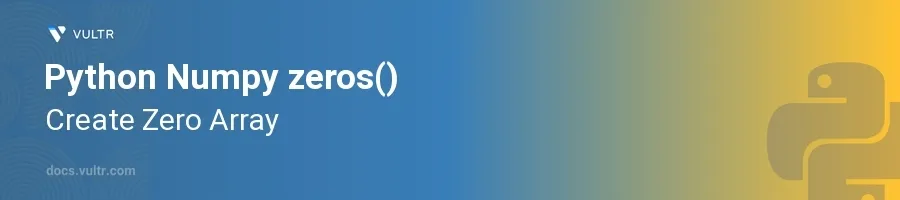
Introduction
The numpy.zeros()
function in Python is a part of the NumPy library, widely known for its scientific computing capabilities. This function quickly generates arrays filled with zero values, which is particularly useful in various applications like initializing matrices, data preprocessing, and setting up default array templates in machine learning algorithms.
In this article, you will learn how to deploy the numpy.zeros()
function effectively in generating zero-filled arrays in Python. Explore how to create arrays of different shapes and dimensions and understand the use of data type specifications to optimize your array constructions.
Understanding the zeros() Function
Creating a Simple Zero Array
Import the NumPy library.
Use
numpy.zeros()
to create an array.pythonimport numpy as np zero_array = np.zeros(5) print(zero_array)
This code snippet creates a 1D array of length 5 filled with zeros. The output will be an array
[0. 0. 0. 0. 0.]
.
Specifying the Array Shape
Specify a shape as a tuple to generate multi-dimensional arrays.
Create a 2D or 3D zero array using this shape specification.
pythontwo_d_array = np.zeros((2, 3)) print(two_d_array) three_d_array = np.zeros((2, 3, 4)) print(three_d_array)
The first
two_d_array
generates a 2D array with 2 rows and 3 columns, all filled with zeros. The secondthree_d_array
shows how to create a 3D array with dimensions 2x3x4.
Data Type Specifications
Using Different Data Types
Understand that the default data type for the zeros array is
float64
.Specify different data types such as
int
,float32
, orcomplex
.pythonint_zeros = np.zeros((3, 3), dtype=int) print(int_zeros) complex_zeros = np.zeros((3, 3), dtype=complex) print(complex_zeros)
Here,
int_zeros
creates a 3x3 array of integers.complex_zeros
makes a 3x3 array capable of holding complex numbers, initialized to zero.
Impact of Data Types on Array Application
Know that using the appropriate data type can reduce memory usage and improve performance.
Choose the data type based on the data that the array will store or the operations it will undergo.
- Arrays intended for integer operations or indices should use
int
. - When working with real numbers in high precision,
float64
is appropriate. - For complex number operations, use
complex
.
- Arrays intended for integer operations or indices should use
This thoughtful selection ensures that the array operations are optimized for both speed and space, which is critical in large-scale computing tasks.
Practical Applications of zeros()
Initialize Arrays for Algorithms
- In many algorithms, particularly in machine learning and image processing, initializing parameters or buffers with zeros is a standard practice.
- Use
numpy.zeros()
to set up these initial states efficiently.
Placeholder Arrays in Data Processing
- When preparing datasets, often a placeholder array is required.
- Utilize
numpy.zeros()
to create these placeholders, ensuring that they have the correct shape and data type for subsequent data manipulation.
Using NumPy's zero-filled arrays as placeholders makes later data insertions and transformations straightforward and error-free, promoting cleaner and more maintainable code.
Conclusion
The numpy.zeros()
function in Python is indispensable for efficiently creating zero-filled arrays, significantly aiding in numerical computation and data preparation tasks. By mastering this function, you enhance your ability to manage array-based data structures and improve the overall performance of scientific computations and algorithm implementations. Employ the techniques discussed here to ensure that arrays are initialized correctly and effectively for any application the situation demands.
No comments yet.